Go – Function as Parameter
Functions in Go are first-class citizens, meaning they can be passed as arguments to other functions, enabling higher-order programming. This is a powerful feature that allows dynamic and reusable code.
In this tutorial, we will discuss how to pass a function as a parameter, the syntax, examples, and benefits of using functions as parameters in Go.
Syntax of Function as Parameter
The syntax for passing a function as a parameter is as follows:
</>
Copy
func higherOrderFunction(paramFunc func(paramType) returnType) {
// Call the passed function
result := paramFunc(argument)
fmt.Println(result)
}
Here:
paramFunc
: The parameter representing the function being passed.paramType
: The type of the parameters the passed function accepts.returnType
: The return type of the passed function.
Examples of Functions as Parameters
Example 1: Passing a Function as Parameter
Here’s an example of passing a function as a parameter:
</>
Copy
package main
import "fmt"
// Function to be passed as a parameter
func square(num int) int {
return num * num
}
// Higher-order function
func processNumber(num int, operation func(int) int) {
result := operation(num)
fmt.Println("Result:", result)
}
func main() {
// Pass the square function as a parameter
processNumber(5, square)
}
Explanation
- Define Function: The function
square
takes an integer and returns its square. - Define Higher-Order Function: The
processNumber
function accepts an integer and a function as parameters. - Call Function: The
square
function is passed toprocessNumber
, which calls it with the given number.
Output
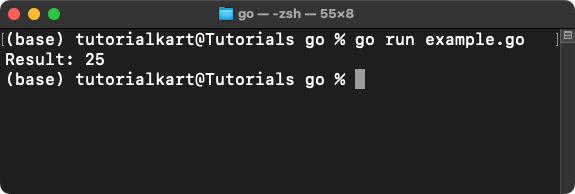
Example 2: Using an Anonymous Function
Instead of defining a separate function, you can use an anonymous function as a parameter:
</>
Copy
package main
import "fmt"
// Higher-order function
func processNumber(num int, operation func(int) int) {
result := operation(num)
fmt.Println("Result:", result)
}
func main() {
// Pass an anonymous function as a parameter
processNumber(10, func(n int) int {
return n * 2
})
}
Explanation
- Define Higher-Order Function: The
processNumber
function takes a number and a function as arguments. - Pass Anonymous Function: An inline anonymous function is passed as the second argument, which doubles the number.
- Call Function: The anonymous function is invoked within
processNumber
.
Output
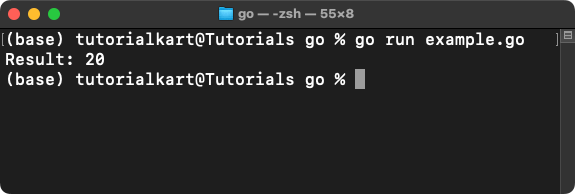
Example 3: Function with Multiple Operations
You can pass multiple functions to apply different operations:
</>
Copy
package main
import "fmt"
// Define operations
func add(a, b int) int {
return a + b
}
func multiply(a, b int) int {
return a * b
}
// Higher-order function
func calculate(a, b int, operation func(int, int) int) {
result := operation(a, b)
fmt.Println("Result:", result)
}
func main() {
calculate(3, 4, add) // Pass add function
calculate(3, 4, multiply) // Pass multiply function
}
Explanation
- Define Operations: Two functions
add
andmultiply
are defined for addition and multiplication. - Define Higher-Order Function: The
calculate
function accepts two integers and a function. - Call Function: Both
add
andmultiply
are passed as arguments tocalculate
for different operations.
Output
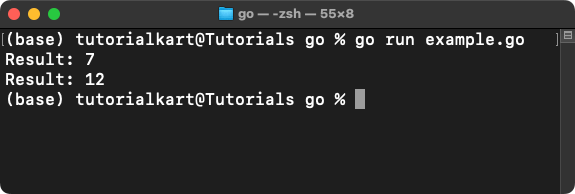
Points to Remember
- Functions are first-class citizens in Go, enabling dynamic and reusable code patterns.
- Passing functions as parameters is useful for implementing callbacks and higher-order programming.
- You can use both named and anonymous functions as parameters.