Go – Function Naming Convention
Proper naming conventions make your code readable, maintainable, and consistent. Go has specific guidelines for naming functions, aligning with its emphasis on simplicity and clarity.
In this tutorial, we will cover these conventions, their importance, and examples to illustrate best practices.
Basic Rules for Function Naming in Go
Go follows these basic rules for naming functions:
- Case Sensitivity: Function names in Go are case-sensitive.
- Exported and Unexported Functions:
- Exported: Functions starting with an uppercase letter are accessible outside their package.
- Unexported: Functions starting with a lowercase letter are private to the package.
- Alphanumeric and Underscore Only: Function names should consist of letters, numbers, and underscores. Special characters are not allowed.
- No Reserved Keywords: Function names cannot use reserved keywords like
if
,for
, orfunc
.
Go Function Naming Guidelines
Here are some best practices for naming functions in Go:
- Use Descriptive Names: Choose names that clearly describe the function’s purpose. For example,
calculateSum
is more descriptive thansum
. - Use CamelCase: Function names should follow the camelCase style, such as
fetchData
orprocessInput
. - Keep Names Short and Clear: Avoid overly long names. For example, use
getUser
instead ofretrieveUserDataFromDatabase
. - Prefix for Action: Start the function name with a verb to indicate its action, such as
fetch
,calculate
, orvalidate
. - Consistency: Maintain consistent naming conventions across your codebase.
Examples of Function Naming
1 Exported Functions
Exported functions start with an uppercase letter and are accessible outside their package:
package main
import "fmt"
// Exported function
func CalculateSum(a int, b int) int {
return a + b
}
func main() {
result := CalculateSum(10, 20)
fmt.Println("Sum:", result)
}
Explanation: The function CalculateSum
starts with an uppercase letter, making it accessible outside the package.
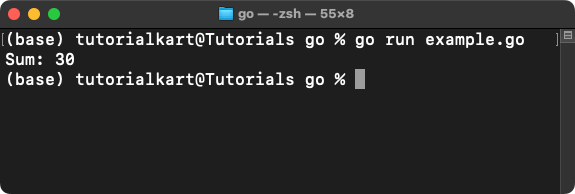
2 Unexported Functions
Unexported functions start with a lowercase letter and are private to their package:
package main
import "fmt"
// Unexported function
func calculateDifference(a int, b int) int {
return a - b
}
func main() {
result := calculateDifference(10, 5)
fmt.Println("Difference:", result)
}
Explanation: The function calculateDifference
starts with a lowercase letter, making it private to the package.
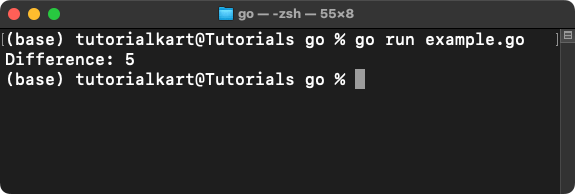
3 Verb-Based Naming
Using a verb-based naming convention makes functions more meaningful:
package main
import "fmt"
// Verb-based naming
func fetchUserData(userID int) string {
return fmt.Sprintf("User data for ID: %d", userID)
}
func main() {
data := fetchUserData(101)
fmt.Println(data)
}
Explanation: The function fetchUserData
clearly indicates its purpose through its name.
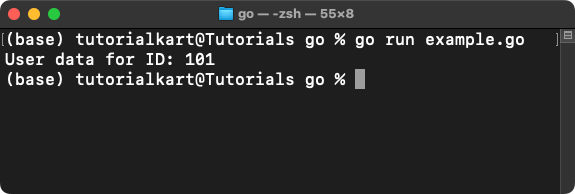
4 Consistent Naming Across Related Functions
Maintain consistency in naming related functions:
package main
import "fmt"
// Related functions with consistent naming
func addNumbers(a int, b int) int {
return a + b
}
func subtractNumbers(a int, b int) int {
return a - b
}
func main() {
fmt.Println("Addition:", addNumbers(5, 3))
fmt.Println("Subtraction:", subtractNumbers(5, 3))
}
Explanation: The functions addNumbers
and subtractNumbers
share consistent naming conventions for clarity and organization.
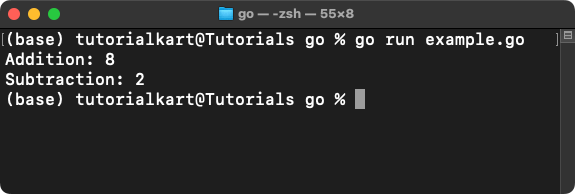
Points to Remember
- Exported vs. Unexported: Use uppercase for exported functions and lowercase for unexported ones.
- Descriptive Names: Use meaningful names that describe the function’s purpose.
- Verb-Based Naming: Start function names with action verbs for clarity.
- Consistency: Maintain consistent naming conventions across the codebase for better readability and maintainability.