Go – Function Overloading
Function overloading is a feature in some programming languages that allows multiple functions to have the same name but different parameters. However, Go does not natively support function overloading. Instead, we can achieve similar behavior using workarounds like variadic functions, interfaces, or custom logic. Let’s dive into how you can mimic function overloading in Go.
What is Function Overloading?
Function overloading allows you to define multiple functions with the same name but different parameter types or numbers. For example:
// This is NOT supported in Go
func add(a int, b int) int {
return a + b
}
func add(a float64, b float64) float64 {
return a + b
}
In Go, such definitions will result in a compilation error because function names must be unique within the same scope.
How to Mimic Function Overloading in Go
Although Go doesn’t support function overloading, you can achieve similar functionality using the following techniques:
- Variadic Functions: Accept a variable number of arguments.
- Empty Interface (
interface{}
): Accept arguments of any type. - Type Switch: Determine the type of arguments at runtime.
1 Using Variadic Functions
A variadic function can take a variable number of arguments, which can be used to mimic function overloading:
package main
import "fmt"
// Variadic function to add integers
func add(numbers ...int) int {
sum := 0
for _, num := range numbers {
sum += num
}
return sum
}
func main() {
fmt.Println("Sum of 1, 2:", add(1, 2)) // Two arguments
fmt.Println("Sum of 1, 2, 3:", add(1, 2, 3)) // Three arguments
}
Explanation
- Define Variadic Function: The function
add
accepts a variable number of integers usingnumbers ...int
. - Iterate Over Arguments: The
for
loop iterates over all arguments to calculate the sum. - Call with Different Arguments: The function is called with varying numbers of arguments to mimic overloading.
Output
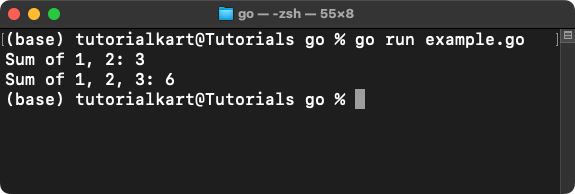
2 Using Empty Interface (interface{}
)
The interface{}
type allows a function to accept arguments of any type. You can use this to handle different types dynamically:
package main
import "fmt"
// Function to handle different argument types
func printValue(value interface{}) {
switch v := value.(type) {
case int:
fmt.Println("Integer:", v)
case string:
fmt.Println("String:", v)
case float64:
fmt.Println("Float:", v)
default:
fmt.Println("Unknown type")
}
}
func main() {
printValue(42) // Integer
printValue("Hello") // String
printValue(3.14) // Float
}
Explanation
- Define Function: The
printValue
function accepts an argument of typeinterface{}
. - Use Type Switch: A
switch
statement checks the type of the argument at runtime. - Call Function: The function is called with arguments of different types.
Output
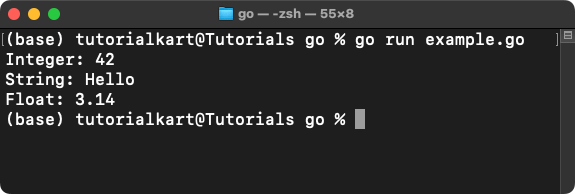
3 Using Custom Logic
You can define different functions with unique names and call the appropriate one based on custom logic:
package main
import "fmt"
// Function for adding integers
func addInts(a int, b int) int {
return a + b
}
// Function for adding floats
func addFloats(a float64, b float64) float64 {
return a + b
}
func main() {
fmt.Println("Sum of integers:", addInts(10, 20))
fmt.Println("Sum of floats:", addFloats(1.5, 2.3))
}
Explanation
- Define Functions: Separate functions
addInts
andaddFloats
are defined for integers and floats, respectively. - Call Functions: The appropriate function is called based on the argument type.
Output
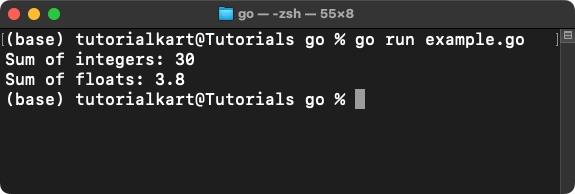
Key Notes
- Go does not natively support function overloading due to its simplicity and focus on explicitness.
- Techniques like variadic functions,
interface{}
, and custom logic can mimic function overloading. - Using clear and descriptive function names is recommended for better readability and maintainability.