Go – Function Pointer
A function pointer is a variable that stores the address of a function, allowing you to pass functions as arguments to other functions or assign them to variables. This enables higher-order functions and dynamic function calls in Go. In this tutorial, we will explore the syntax, usage, and examples to understand this concept.
What is a Function Pointer?
In Go, functions are first-class citizens, meaning you can:
- Assign functions to variables.
- Pass functions as arguments to other functions.
- Return functions from other functions.
A function pointer allows you to store the address of a function in a variable, enabling dynamic function calls and functional programming paradigms.
Example Syntax for Function Pointers
</>
Copy
func functionName(parameters) returnType {
// Function body
}
// Assign the function to a variable
var functionPointer = functionName
// Use the function pointer to call the function
functionPointer(arguments)
Examples of Function Pointers
Example 1: Assigning a Function to a Variable
Here’s an example of assigning a function to a variable and using the variable to call the function:
</>
Copy
package main
import "fmt"
// Define a function
func greet(name string) {
fmt.Println("Hello,", name)
}
func main() {
// Assign the function to a variable
greetPointer := greet
// Call the function using the pointer
greetPointer("World")
}
Explanation
- Define Function: A function
greet
is defined, which takes a string parameter and prints a greeting message. - Assign to Variable: The function
greet
is assigned to the variablegreetPointer
. - Call Function: The function is called using
greetPointer
, behaving the same as callinggreet
directly.
Output
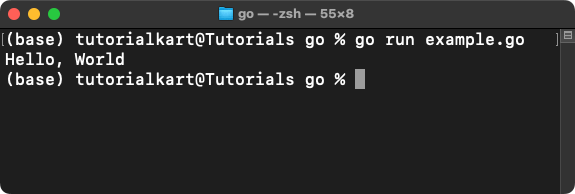
Example 2: Passing a Function as an Argument
You can pass a function as an argument to another function:
</>
Copy
package main
import "fmt"
// Define a function to be passed
func add(a int, b int) int {
return a + b
}
// Define a higher-order function
func calculate(a int, b int, operation func(int, int) int) {
result := operation(a, b)
fmt.Println("Result:", result)
}
func main() {
// Pass the add function as an argument
calculate(10, 20, add)
}
Explanation
- Define Add Function: The
add
function takes two integers, adds them, and returns the sum. - Define Higher-Order Function: The
calculate
function accepts two integers and a function as arguments. - Call Higher-Order Function: The
add
function is passed as an argument tocalculate
, which calls it to compute the result.
Output
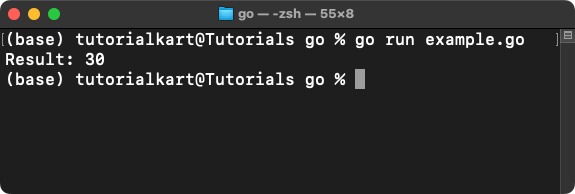
Example 3: Returning a Function
A function can return another function:
</>
Copy
package main
import "fmt"
// Function returning another function
func multiplier(factor int) func(int) int {
return func(num int) int {
return num * factor
}
}
func main() {
double := multiplier(2) // Returns a function that doubles numbers
triple := multiplier(3) // Returns a function that triples numbers
fmt.Println("Double of 5:", double(5))
fmt.Println("Triple of 5:", triple(5))
}
Explanation
- Define Function: The
multiplier
function takes an integer and returns another function. - Inner Function: The returned function multiplies its input by the
factor
. - Call Returned Function: Functions
double
andtriple
are created and used to compute results.
Output
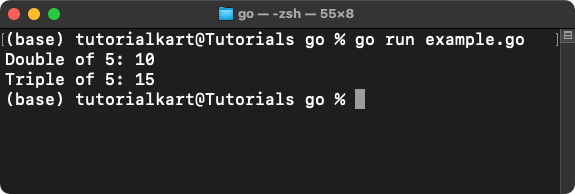
Key Notes
- Functions in Go are first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
- Using function pointers allows for dynamic and reusable code.
- Higher-order functions enable functional programming paradigms in Go.