Go – Function Return
In this tutorial, we will explore how to use return statements in functions in the Go programming language (Golang). Functions in Go can return values to the caller using the return
keyword. Go supports single, multiple, and named return values, making functions versatile and expressive. We will cover these variations with practical examples and detailed explanations.
Basic Syntax for Return
The basic syntax for a function that returns a value is:
</>
Copy
func functionName(parameters) returnType {
// Function body
return value
}
Here:
returnType
: The type of the value the function returns.return
: The keyword used to return a value from the function.value
: The value or expression that is returned.
Examples of Function Returns
Example 1: Single Return Value
A simple function that returns a single value:
</>
Copy
package main
import "fmt"
// Function that returns the square of a number
func square(num int) int {
return num * num
}
func main() {
result := square(5) // Call the function
fmt.Println("Square of 5:", result)
}
Explanation
- Define Function: The
square
function accepts an integer parameter and returns its square. - Use Return Statement: The product
num * num
is returned using thereturn
keyword. - Call Function: The result of
square(5)
is stored inresult
and printed.
Output
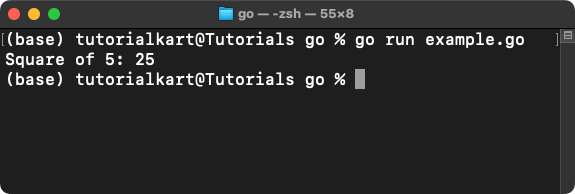
Example 2: Multiple Return Values
Go functions can return multiple values:
</>
Copy
package main
import "fmt"
// Function that returns the quotient and remainder of a division
func divide(a int, b int) (int, int) {
return a / b, a % b
}
func main() {
quotient, remainder := divide(10, 3) // Call the function
fmt.Println("Quotient:", quotient)
fmt.Println("Remainder:", remainder)
}
Explanation
- Define Function: The
divide
function accepts two integers and returns two values: quotient and remainder. - Use Multiple Returns: Both
a / b
anda % b
are returned separated by commas. - Capture Results: The results are stored in
quotient
andremainder
using multiple assignments.
Output
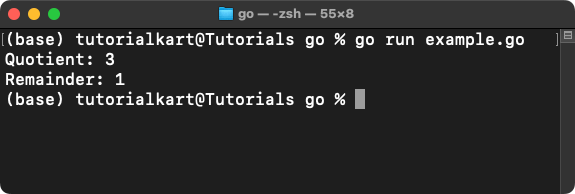
Example 3: Named Return Values
Go allows naming return values in the function signature:
</>
Copy
package main
import "fmt"
// Function with named return values
func rectangleDimensions(length, width int) (area, perimeter int) {
area = length * width
perimeter = 2 * (length + width)
return // Named return values are automatically returned
}
func main() {
area, perimeter := rectangleDimensions(5, 3) // Call the function
fmt.Println("Area:", area)
fmt.Println("Perimeter:", perimeter)
}
Explanation
- Define Named Return Values: The function
rectangleDimensions
definesarea
andperimeter
as named return values. - Assign Values: The values for
area
andperimeter
are computed inside the function. - Automatic Return: Named return values are automatically returned when the
return
keyword is used without arguments.
Output
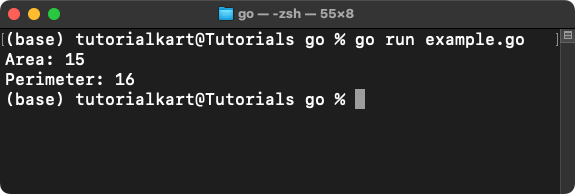
Example 4: Returning a Function
A function can return another function:
</>
Copy
package main
import "fmt"
// Function that returns another function
func multiplier(factor int) func(int) int {
return func(num int) int {
return num * factor
}
}
func main() {
double := multiplier(2) // Returns a function that doubles numbers
fmt.Println("Double of 5:", double(5))
}
Explanation
- Define Function: The
multiplier
function returns an anonymous function. - Return a Function: The returned function takes an integer and multiplies it by
factor
. - Call Returned Function: The returned function is assigned to
double
and invoked with an argument.
Output
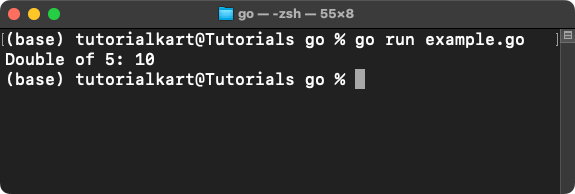
Important Points
- A function can return a single value, multiple values, or no value.
- Named return values improve code readability and reduce the need for explicit return statements.
- Returning functions allows creating higher-order functions for advanced use cases.