Go – Slice Without Last Element
In Go, slices are dynamic collections, and you can easily create a new slice that excludes the last element by using slicing operations. This operation does not modify the original slice but creates a new view of the slice without its last element.
In this tutorial, we will explore how to exclude the last element from a slice in Go with practical examples and detailed explanations.
Steps to Create a Slice Without the Last Element
- Validate the Slice Length: Ensure that the slice is not empty to avoid runtime errors.
- Use Slicing: Use the syntax
slice[:len(slice)-1]
to exclude the last element. - Preserve the Original Slice: The operation creates a new slice and does not modify the original slice.
Examples of Slices Without the Last Element
1. Remove the Last Element from a Slice of Integers
This example demonstrates how to exclude the last element from a slice of integers:
</>
Copy
package main
import "fmt"
// Function to create a slice without the last element
func sliceWithoutLast(slice []int) []int {
if len(slice) == 0 {
return slice // Return the original slice if it's empty
}
return slice[:len(slice)-1]
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Get the slice without the last element
updatedSlice := sliceWithoutLast(numbers)
// Print the original and updated slices
fmt.Println("Original Slice:", numbers)
fmt.Println("Slice Without Last Element:", updatedSlice)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns it unchanged if true.
- Create New Slice: The new slice is created using
slice[:len(slice)-1]
, which excludes the last element. - Print Results: The original and updated slices are printed to demonstrate the result.
Output
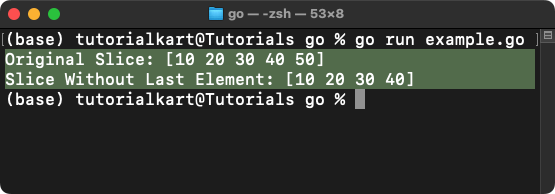
2. Remove the Last Element from a Slice of Strings
This example demonstrates how to exclude the last element from a slice of strings:
</>
Copy
package main
import "fmt"
// Function to create a slice without the last element
func sliceWithoutLast(slice []string) []string {
if len(slice) == 0 {
return slice // Return the original slice if it's empty
}
return slice[:len(slice)-1]
}
func main() {
// Declare and initialize a slice
words := []string{"apple", "banana", "cherry", "date"}
// Get the slice without the last element
updatedSlice := sliceWithoutLast(words)
// Print the original and updated slices
fmt.Println("Original Slice:", words)
fmt.Println("Slice Without Last Element:", updatedSlice)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns it unchanged if true.
- Create New Slice: The new slice is created using
slice[:len(slice)-1]
, which excludes the last element. - Print Results: The original and updated slices are printed to demonstrate the result.
Output
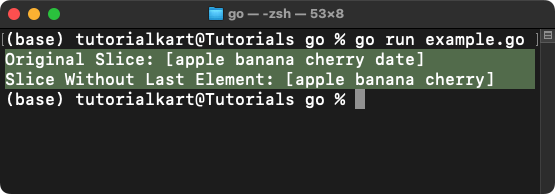
Points to Remember
- Slicing Syntax: Use
slice[:len(slice)-1]
to exclude the last element from a slice. - Bounds Checking: Always check if the slice is not empty before slicing to avoid runtime errors.
- Original Slice Unchanged: The original slice remains unchanged, and the operation creates a new slice referencing the same underlying array.
- Generic Usage: This technique can be applied to slices of any type, such as integers, strings, or custom structs.