Go – Infinite For Loop
An infinite loop is a loop that runs indefinitely unless explicitly terminated. Infinite loops are useful for scenarios such as waiting for events, continuously checking conditions, or running servers.
In this tutorial, we will go through how to implement and control infinite loops with examples.
Syntax of Infinite For Loop
The syntax of an infinite for
loop in Go is simple. You omit all three components (initialization, condition, and post statement):
for {
// Code to execute infinitely
}
This creates a loop that will run indefinitely until explicitly terminated using break
or the program is interrupted.
Example 1: Simple Infinite Loop
Here is an example of a simple infinite loop:
package main
import "fmt"
func main() {
for {
fmt.Println("This loop will run forever!")
}
}
Output
Controlling an Infinite Loop
Infinite loops can be controlled using break
to exit the loop or continue
to skip to the next iteration. Here’s an example:
package main
import "fmt"
func main() {
count := 0
for {
fmt.Println("Iteration:", count)
count++
// Exit the loop when count reaches 5
if count == 5 {
break
}
}
fmt.Println("Loop terminated.")
}
Output
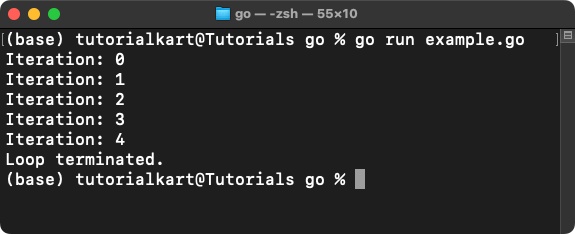
Example 2: Infinite Loop with Continue
The continue
statement can be used to skip the rest of the current iteration and start the next iteration:
package main
import "fmt"
func main() {
count := 0
for {
count++
// Skip even numbers
if count%2 == 0 {
continue
}
fmt.Println("Odd number:", count)
// Exit the loop when count exceeds 10
if count > 10 {
break
}
}
}
Output
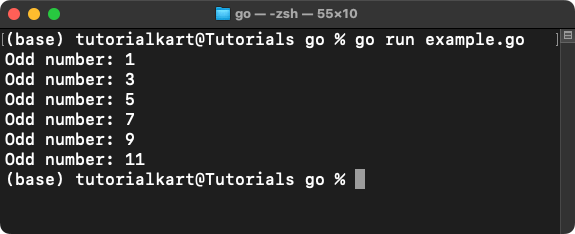
When to Use Infinite Loops
- Continuously monitoring for events, such as in servers or event listeners.
- Running tasks repeatedly without a predefined end condition.
- Handling input in interactive programs or games.
Conclusion
Infinite for
loops in Go are simple to implement and powerful for scenarios requiring continuous execution. Using break
and continue
, you can control the flow of the loop to suit your program’s needs. Ensure that loops are designed with proper termination conditions to avoid unintended infinite execution.