Go – Largest of Three Numbers
In this tutorial, we will learn how to find the largest of three numbers using the Go programming language (Golang). Finding the largest number among three inputs is a basic problem-solving task that demonstrates decision-making in programming. We will use conditional statements to implement this logic and provide a detailed example program.
Logic to Find the Largest of Three Numbers
The logic for finding the largest of three numbers involves comparing the three numbers using conditional statements:
- Compare the First Two Numbers: Check if the first number is greater than or equal to the second.
- Compare the Result with the Third Number: If the first number is greater than the second, compare it with the third number. Otherwise, compare the second number with the third.
- Determine the Largest: The largest number is the result of these comparisons.
Example Program to Find the Largest of Three Numbers
In this example, we will take three numbers, use logical operators and conditional statements, to find largest of the three numbers.
Program – example.go
</>
Copy
package main
import "fmt"
func main() {
// Declare variables to hold three numbers
var num1, num2, num3 int
// Prompt the user to enter three numbers
fmt.Print("Enter three integers separated by space: ")
fmt.Scan(&num1, &num2, &num3)
// Find the largest number
var largest int
if num1 >= num2 && num1 >= num3 {
largest = num1
} else if num2 >= num1 && num2 >= num3 {
largest = num2
} else {
largest = num3
}
// Print the largest number
fmt.Println("The largest number is:", largest)
}
Explanation of Program
- Declare Variables: Three integer variables,
num1
,num2
, andnum3
, are declared to store the user input. - Prompt the User: The program prompts the user to enter three integers separated by spaces.
- Read the Inputs: The
fmt.Scan
function reads the inputs and stores them in the declared variables. - Find the Largest: Conditional statements are used to compare the three numbers. The variable
largest
stores the largest number based on the comparisons. - Print the Largest: The program prints the largest number to the console.
Output
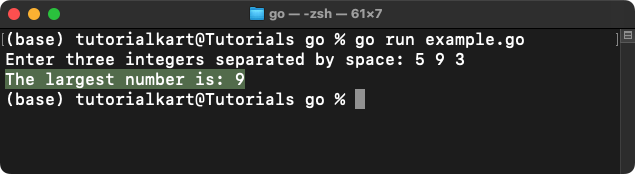
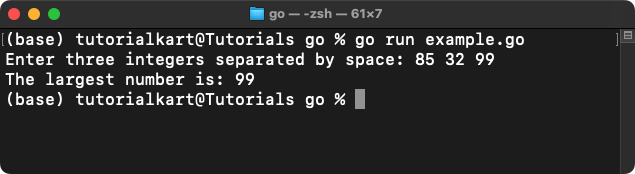
This program efficiently finds the largest number among three inputs using conditional statements and displays the result.