Go – Logical Operators
Logical operators are used to combine conditional statements and evaluate boolean expressions. These operators are essential for decision-making and controlling the flow of a program.
In this tutorial, we will explore the available logical operators in Go along with practical examples and detailed explanations.
List of Logical Operators
Go provides the following logical operators:
Operator | Description | Example | Result |
---|---|---|---|
&& | Logical AND | a && b | true if both a and b are true |
|| | Logical OR | a || b | true if either a or b is true |
! | Logical NOT | !a | true if a is false |
Examples of Logical Operators
Let’s explore how to use these logical operators in a Go program.
Example 1: Logical AND (&&
)
The &&
operator returns true
only if both conditions are true
. Here’s an example:
</>
Copy
package main
import "fmt"
func main() {
a := true
b := false
fmt.Println("a && b:", a && b) // false
fmt.Println("true && true:", true && true) // true
}
Explanation
- Declare Variables: Two boolean variables
a
andb
are initialized astrue
andfalse
, respectively. - Evaluate Logical AND: The expression
a && b
returnsfalse
becauseb
isfalse
. - Print Results: The results of logical operations are printed using
fmt.Println
.
Output
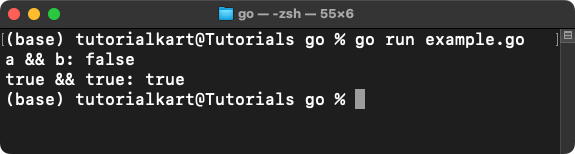
Example 2: Logical OR (||
)
The ||
operator returns true
if at least one condition is true
. Here’s an example:
</>
Copy
package main
import "fmt"
func main() {
a := true
b := false
fmt.Println("a || b:", a || b) // true
fmt.Println("false || false:", false || false) // false
}
Explanation
- Declare Variables: Two boolean variables
a
andb
are initialized astrue
andfalse
, respectively. - Evaluate Logical OR: The expression
a || b
returnstrue
becausea
istrue
. - Print Results: The results of logical operations are printed using
fmt.Println
.
Output
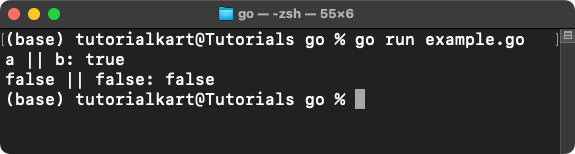
Example 3: Logical NOT (!
)
The !
operator reverses the boolean value. Here’s an example:
</>
Copy
package main
import "fmt"
func main() {
a := true
fmt.Println("!a:", !a) // false
fmt.Println("!false:", !false) // true
}
Explanation
- Declare Variable: A boolean variable
a
is initialized astrue
. - Evaluate Logical NOT: The expression
!a
returnsfalse
becausea
istrue
. - Print Results: The result of the NOT operation is printed using
fmt.Println
.
Output
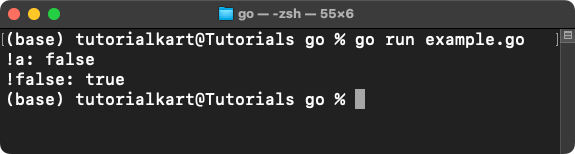
Key Notes for Logical Operators
- The
&&
operator evaluates totrue
only if both conditions aretrue
. - The
||
operator evaluates totrue
if at least one condition istrue
. - The
!
operator inverts the boolean value. - Logical operators are often used in
if
statements to control program flow.