Go – Nested For Loop
A nested for
loop is a loop inside another loop. These are useful when dealing with multidimensional data structures, creating patterns, or performing repetitive tasks within repetitive tasks.
In this tutorial, we will go through examples with detailed explanations to help you understand and implement nested for
loops.
Syntax of Nested For Loops
The syntax of a nested for
loop involves placing one for
loop inside another:
for initialization; condition; post {
for initialization; condition; post {
// Code to execute in the inner loop
}
// Code to execute in the outer loop
}
The outer loop runs for each iteration of its condition, while the inner loop runs fully for every iteration of the outer loop.
Example 1: Printing a Multiplication Table using Nested For Loop
Let’s create a program to print a multiplication table using nested for
loops:
package main
import "fmt"
func main() {
// Outer loop for rows
for i := 1; i <= 5; i++ {
// Inner loop for columns
for j := 1; j <= 5; j++ {
fmt.Printf("%d\t", i*j)
}
fmt.Println() // New line after each row
}
}
Explanation
- Outer Loop: The outer loop iterates from 1 to 5, representing the rows of the multiplication table.
- Inner Loop: The inner loop iterates from 1 to 5 for each iteration of the outer loop, representing the columns.
- Multiplication: The product of the current row and column index is calculated and printed using
fmt.Printf
. - New Line: After the inner loop completes, a new line is added using
fmt.Println()
to separate rows.
Output
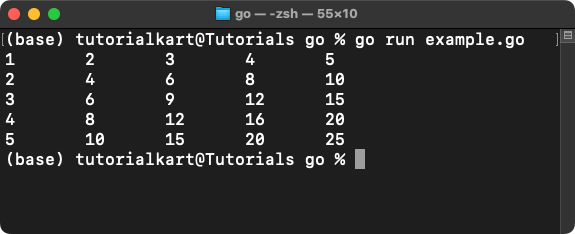
Example 2: Creating a Pattern using Nested For Loop
Nested loops are useful for creating patterns. Here’s an example of printing a triangle pattern:
package main
import "fmt"
func main() {
// Outer loop for rows
for i := 1; i <= 5; i++ {
// Inner loop for columns
for j := 1; j <= i; j++ {
fmt.Print("*")
}
fmt.Println() // New line after each row
}
}
Explanation
- Outer Loop: The outer loop runs from 1 to 5, representing the number of rows.
- Inner Loop: The inner loop prints asterisks (*) for each column in the current row, determined by the value of
i
. - New Line: After printing the asterisks for a row, a new line is added using
fmt.Println()
.
Output
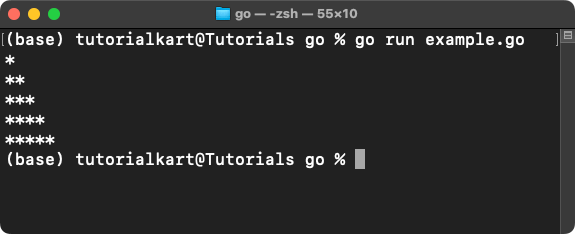
Conclusion
Nested for
loops in Go allow you to handle multidimensional data and create patterns. By understanding how the inner and outer loops interact, you can efficiently solve a variety of programming problems.