Go Operators
In this tutorial, we will learn about the different types of operators in the Go programming language (Golang). Operators are special symbols or keywords used to perform operations on variables and values. Go provides a rich set of operators to work with arithmetic, logical, relational, and other operations. We will explore these operators with examples for better understanding.
Types of Operators in Go
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Miscellaneous Operators
1 Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations:
Operator | Description |
---|---|
+ | Addition |
- | Subtraction |
* | Multiplication |
/ | Division |
% | Modulus (remainder) |
Example
package main
import "fmt"
func main() {
a := 10
b := 3
fmt.Println("Addition:", a+b)
fmt.Println("Subtraction:", a-b)
fmt.Println("Multiplication:", a*b)
fmt.Println("Division:", a/b)
fmt.Println("Modulus:", a%b)
}
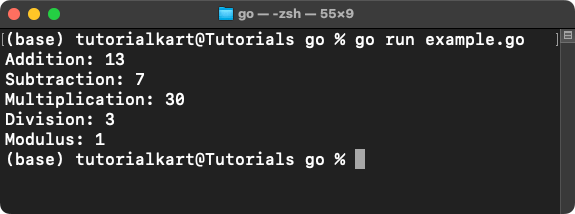
Arithmetic Operators
- Go – Addition Operator
- Go – Subtraction Operator
- Go – Multiplication Operator
- Go – Division Operator
- Go – Remainder Operator
- Go – Increment Operator
- Go – Decrement Operator
2 Relational Operators
Relational operators are used to compare two values and return a boolean result:
Operator | Description |
---|---|
== | Equal to |
!= | Not equal to |
> | Greater than |
< | Less than |
>= | Greater than or equal to |
<= | Less than or equal to |
Example
package main
import "fmt"
func main() {
a := 10
b := 20
fmt.Println("a == b:", a == b)
fmt.Println("a != b:", a != b)
fmt.Println("a > b:", a > b)
fmt.Println("a < b:", a < b)
fmt.Println("a >= b:", a >= b)
fmt.Println("a <= b:", a <= b)
}
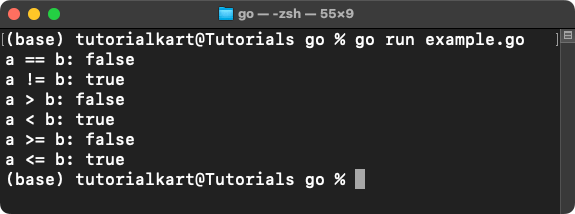
3 Logical Operators
Logical operators are used to combine conditional statements:
Operator | Description |
---|---|
&& | Logical AND |
|| | Logical OR |
! | Logical NOT |
Example
package main
import "fmt"
func main() {
a := true
b := false
fmt.Println("a && b:", a && b)
fmt.Println("a || b:", a || b)
fmt.Println("!a:", !a)
}
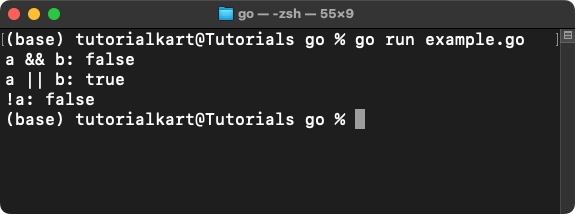
Logical Operators
4 Bitwise Operators
Bitwise operators perform bit-level operations:
Operator | Description |
---|---|
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise XOR |
<< | Left shift |
>> | Right shift |
Example
package main
import "fmt"
func main() {
a := 5 // Binary: 0101
b := 3 // Binary: 0011
fmt.Println("a & b:", a&b)
fmt.Println("a | b:", a|b)
fmt.Println("a ^ b:", a^b)
fmt.Println("a << 1:", a<<1)
fmt.Println("a >> 1:", a>>1)
}
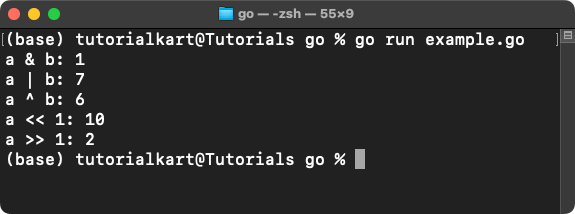
5 Assignment Operators
Assignment operators are used to assign values to variables:
Operator | Description |
---|---|
= | Assign |
+= | Add and assign |
-= | Subtract and assign |
*= | Multiply and assign |
/= | Divide and assign |
%= | Modulus and assign |
Example
package main
import "fmt"
func main() {
a := 10
a += 5
fmt.Println("a += 5:", a)
a -= 3
fmt.Println("a -= 3:", a)
a *= 2
fmt.Println("a *= 2:", a)
a /= 4
fmt.Println("a /= 4:", a)
a %= 3
fmt.Println("a %= 3:", a)
}
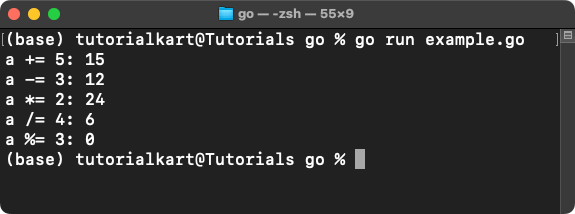
6 Miscellaneous Operators
Operator | Description |
---|---|
& | Address of a variable |
* | Pointer to a variable |
Conclusion
Operators are fundamental to any programming language, and Go provides a rich set of operators to perform a wide range of operations. Understanding these operators will help you write efficient and concise code in your programs.