Go – Palindrome String
A palindrome is a word, phrase, number, or other sequence of characters that reads the same backward as forward, ignoring spaces, punctuation, and capitalization. In this tutorial, we will learn how to determine whether a string is a palindrome using the Go programming language (Golang).
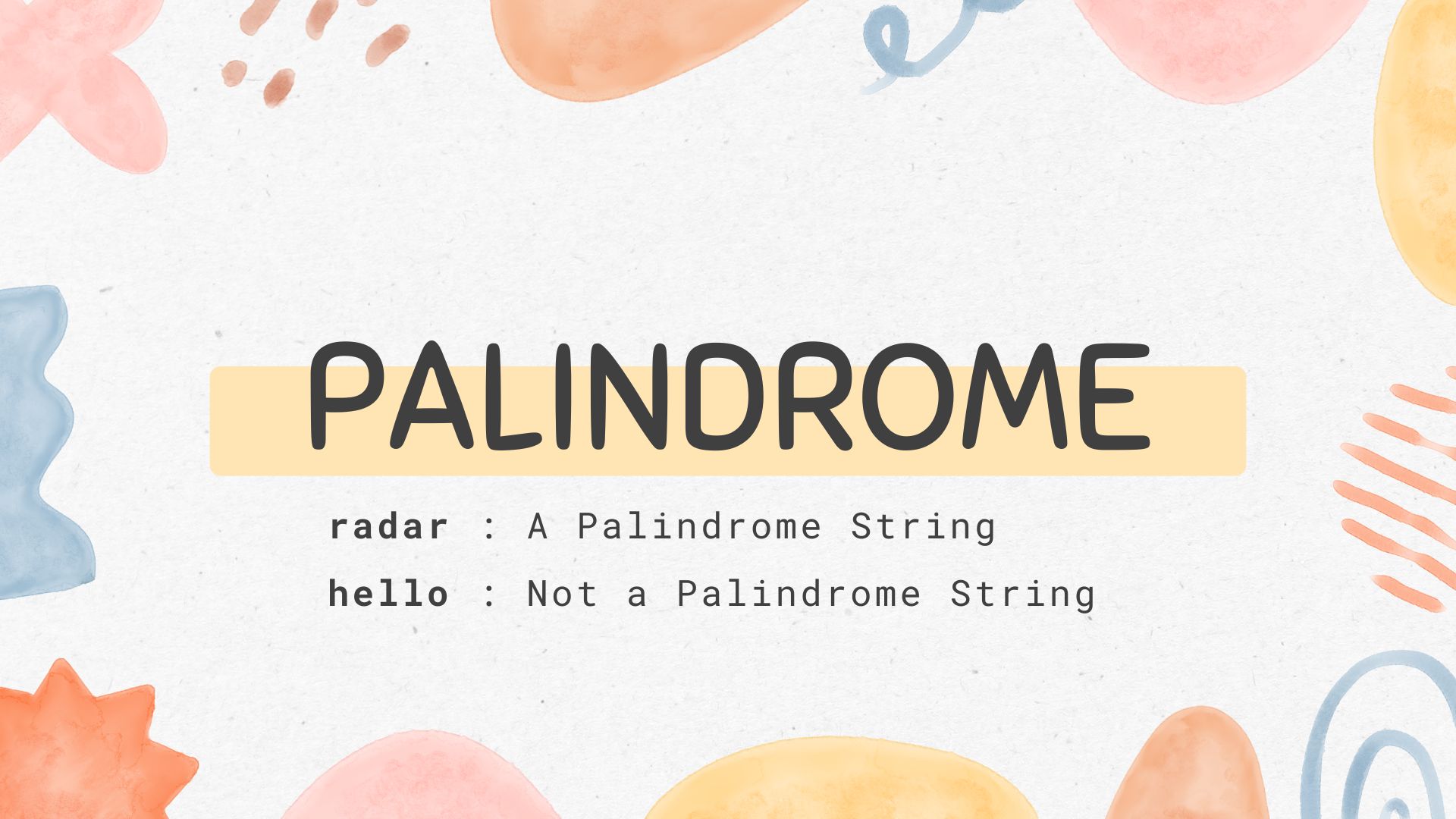
We will explain the logic and provide a detailed example program to find if the given string is a Palindrome or not.
What is a Palindrome String?
A palindrome string is a sequence of characters that remains unchanged when reversed. For example:
- Palindrome Strings: “radar”, “madam”, “level”
- Non-Palindrome Strings: “hello”, “world”
Logic to Check Palindrome String
To check if a string is a palindrome:
- Read the string as input.
- Reverse the string.
- Compare the original string with the reversed string.
- If both strings are identical, it is a palindrome; otherwise, it is not.
Example Program to Check Palindrome String
Program – example.go
</>
Copy
package main
import (
"fmt"
"strings"
)
// Function to check if a string is a palindrome
func isPalindrome(str string) bool {
// Convert string to lowercase to handle case-insensitive comparison
str = strings.ToLower(str)
// Initialize two pointers: one at the start and one at the end
start, end := 0, len(str)-1
// Compare characters from both ends
for start < end {
if str[start] != str[end] {
return false
}
start++
end--
}
return true
}
func main() {
// Input string
var input string
fmt.Print("Enter a string: ")
fmt.Scanln(&input)
// Check if the string is a palindrome
if isPalindrome(input) {
fmt.Println("\"" + input + "\" is a palindrome.")
} else {
fmt.Println("\"" + input + "\" is not a palindrome.")
}
}
Explanation of Program
- Convert to Lowercase: The input string is converted to lowercase using
strings.ToLower
to ensure a case-insensitive comparison. - Two-Pointer Technique: Two pointers are initialized:
start
at the beginning of the string andend
at the last character. - Character Comparison: Characters at
start
andend
are compared. If they differ, the function returnsfalse
. If they match, the pointers are moved inward. - Palindrome Check: If all characters match while traversing, the function returns
true
, indicating the string is a palindrome. - User Input and Output: The user enters a string, which is checked using the
isPalindrome
function, and the result is displayed.
Output
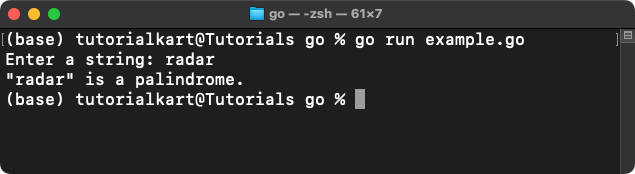
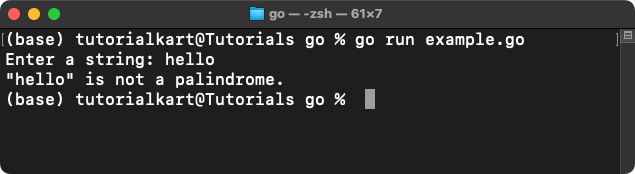
This program demonstrates an efficient way to check whether a string is a palindrome using the two-pointer technique.