Go – Read Float Input from Console
In this tutorial, we will learn how to read float input from the console in the Go programming language (Golang). Accepting floating-point input from the user is a common task in programs that require numerical computation. We’ll explore how to use the fmt.Scan
function and provide a detailed example program.
Syntax to Read Float Input
The basic syntax to read a float input in Go is:
fmt.Scan(&variable)
Here, variable
is a float variable where the input from the console will be stored.
The ampersand (&
) is used to pass the memory address of the variable to the Scan
function.
Example 1: Program to Read Float Input from Console
In this example, we will read a floating point number from console input, and print the same to output.
Program – example.go
package main
import "fmt"
func main() {
// Declare a float variable to hold user input
var input float64
// Prompt the user
fmt.Print("Enter a floating-point number: ")
// Read input from the console
fmt.Scan(&input)
// Print the input back to the console
fmt.Println("You entered:", input)
}
Explanation of Program
- Declare a Variable: A variable of type
float64
is declared to store the user input. - Prompt the User: The
fmt.Print
function is used to display a message prompting the user to enter a floating-point number. - Read the Input: The
fmt.Scan
function reads the input typed by the user and stores it in the variable. The&
operator is used to pass the address of the variable. - Print the Input: The entered floating-point number is printed back to the console using the
fmt.Println
function.
Output
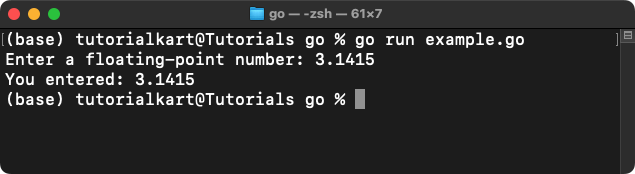
This example demonstrates how to read a floating-point input from the user via the console and display it back.
Example 2: Read Multiple Float Inputs from Console
To read multiple float inputs in a single line, you can use the fmt.Scan
function with multiple variables. Here’s an example:
Program – example.go
package main
import "fmt"
func main() {
// Declare variables to hold user input
var num1, num2 float64
// Prompt the user
fmt.Print("Enter two floating-point numbers separated by space: ")
// Read input from the console
fmt.Scan(&num1, &num2)
// Print the inputs back to the console
fmt.Println("You entered:", num1, "and", num2)
// Print their sum
fmt.Println("Sum:", num1 + num2)
}
- Declare Variables: Two float variables,
num1
andnum2
, are declared. - Prompt the User: The program prompts the user to enter two floating-point numbers separated by a space.
- Read Inputs: The
fmt.Scan
function reads the inputs and stores them in the respective variables. - Print the Inputs: The entered floating-point numbers are displayed back to the console.
Output
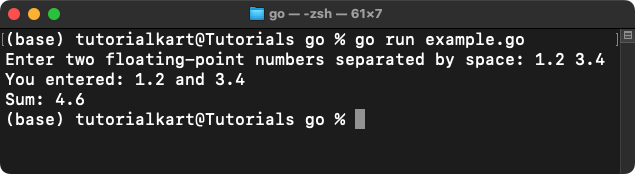
This example demonstrates how to read multiple floating-point inputs from a single input line.