Go – Read Integer Input from Console
In this tutorial, we will learn how to read integer input from the console in the Go programming language (Golang). Accepting numeric input from the user is a common task in interactive programs. We’ll explore a simple method using the fmt.Scan
function and provide a detailed explanation along with example programs.
Syntax to Read Integer Input
The basic syntax to read an integer input in Go is:
fmt.Scan(&variable)
Here, variable
is an integer variable where the input from the console will be stored.
The ampersand (&
) is used to pass the memory address of the variable to the Scan
function.
Example Program to Read Integer Input
Program – read_integer.go
package main
import "fmt"
func main() {
// Declare an integer variable to hold user input
var input int
// Prompt the user
fmt.Print("Enter an integer: ")
// Read input from the console
fmt.Scan(&input)
// Print the input back to the console
fmt.Println("You entered:", input)
}
Explanation of Program
- Declare a Variable: A variable of type
int
is declared to store the user input. - Prompt the User: The
fmt.Print
function is used to display a message prompting the user to enter an integer. - Read the Input: The
fmt.Scan
function reads the input typed by the user and stores it in the variable. The&
operator is used to pass the address of the variable. - Print the Input: The entered integer is printed back to the console using the
fmt.Println
function.
Output
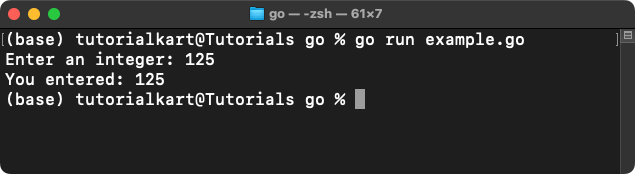
This example demonstrates how to read an integer input from the user via the console and display it back.
Example with Multiple Integers
To read multiple integers in a single input, you can use the fmt.Scan
function with multiple variables. Here’s an example:
Program – read_multiple_integers.go
package main
import "fmt"
func main() {
// Declare variables to hold user input
var num1, num2 int
// Prompt the user
fmt.Print("Enter two integers separated by space: ")
// Read input from the console
fmt.Scan(&num1, &num2)
// Print the inputs back to the console
fmt.Println("You entered:", num1, "and", num2)
// Print their sum
fmt.Println("Sum:", num1 + num2)
}
Explanation of Program
- Declare Variables: Two integer variables,
num1
andnum2
, are declared. - Prompt the User: The program prompts the user to enter two integers separated by a space.
- Read Inputs: The
fmt.Scan
function reads the inputs and stores them in the respective variables. - Print the Inputs: The entered integers are displayed back to the console.
Output
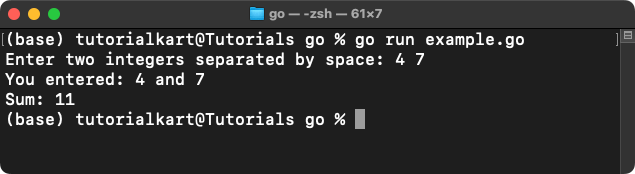
This example demonstrates how to read multiple integers from a single input line.