Go – Relational Operators
Relational operators are used to compare two values, resulting in a boolean value: true
or false
. These operators are commonly used in conditional statements to control the flow of a program.
In this tutorial, we will explore each relational operator in Go with examples and detailed explanations.
List of Relational Operators
The following table lists all relational operators in Go:
Operator | Description | Example | Result |
---|---|---|---|
== | Equal to | a == b | true if a is equal to b |
!= | Not equal to | a != b | true if a is not equal to b |
> | Greater than | a > b | true if a is greater than b |
< | Less than | a < b | true if a is less than b |
>= | Greater than or equal to | a >= b | true if a is greater than or equal to b |
<= | Less than or equal to | a <= b | true if a is less than or equal to b |
Examples of Relational Operators
Let’s explore how to use relational operators in a Go program.
Example 1: Using Relational Operators with Integers
Here’s a simple program to demonstrate relational operators with integers:
</>
Copy
package main
import "fmt"
func main() {
a := 10
b := 20
fmt.Println("a == b:", a == b) // false
fmt.Println("a != b:", a != b) // true
fmt.Println("a > b:", a > b) // false
fmt.Println("a < b:", a < b) // true
fmt.Println("a >= b:", a >= b) // false
fmt.Println("a <= b:", a <= b) // true
}
Explanation
- Declare Variables: Two integer variables,
a
andb
, are initialized with the values 10 and 20, respectively. - Use Relational Operators: Each relational operator is applied to compare
a
andb
. - Print Results: The results of the comparisons are printed using
fmt.Println
.
Output
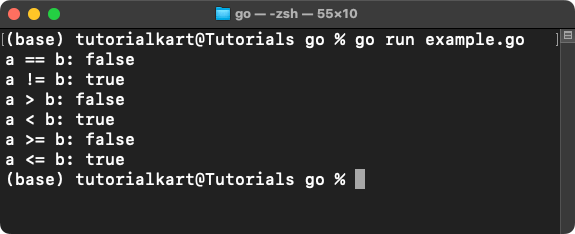
Example 2: Using Relational Operators with Floating-Point Numbers
Relational operators can also be used with floating-point numbers:
</>
Copy
package main
import "fmt"
func main() {
x := 15.5
y := 10.2
fmt.Println("x == y:", x == y) // false
fmt.Println("x != y:", x != y) // true
fmt.Println("x > y:", x > y) // true
fmt.Println("x < y:", x < y) // false
fmt.Println("x >= y:", x >= y) // true
fmt.Println("x <= y:", x <= y) // false
}
Explanation
- Declare Variables: Two floating-point variables,
x
andy
, are initialized with the values 15.5 and 10.2, respectively. - Use Relational Operators: Each relational operator is applied to compare
x
andy
. - Print Results: The results of the comparisons are printed using
fmt.Println
.
Output
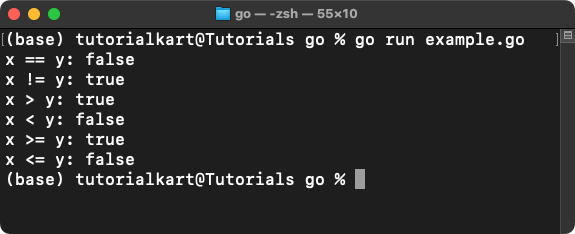
Example 3: Using Relational Operators with Strings
Relational operators can also compare strings lexicographically (dictionary order):
</>
Copy
package main
import "fmt"
func main() {
str1 := "Golang"
str2 := "Go"
fmt.Println("str1 == str2:", str1 == str2) // false
fmt.Println("str1 != str2:", str1 != str2) // true
fmt.Println("str1 > str2:", str1 > str2) // true
fmt.Println("str1 < str2:", str1 < str2) // false
}
Explanation
- Declare Variables: Two string variables,
str1
andstr2
, are initialized with the values"Golang"
and"Go"
, respectively. - Use Relational Operators: Relational operators are applied to compare the strings lexicographically.
- Print Results: The results of the comparisons are printed using
fmt.Println
.
Output
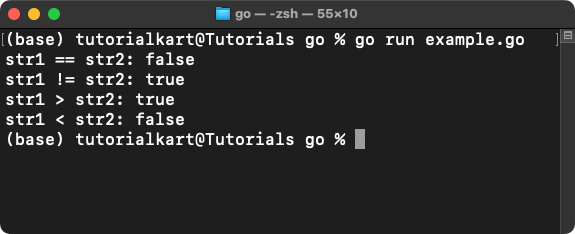
Key Notes for Relational Operators
- Relational operators return a boolean value:
true
orfalse
. - They work with integers, floating-point numbers, and strings.
- String comparisons are case-sensitive and based on lexicographical order.