Go – Reverse a Number
In this tutorial, we will learn how to reverse a number using the Go programming language (Golang). Reversing a number involves rearranging its digits in reverse order. This task demonstrates the use of loops, arithmetic operators, and conditionals in Go. We will provide a step-by-step explanation and a program to achieve this functionality.
Logic to Reverse a Number
The logic to reverse a number involves extracting its digits and rearranging them in reverse order:
- Extract the Last Digit: Use the modulus operator (
%
) to get the last digit of the number. - Build the Reversed Number: Multiply the reversed number by 10 and add the extracted digit to it.
- Remove the Last Digit: Use integer division (
/
) to remove the last digit of the original number. - Repeat: Continue the process until all digits of the number are reversed.
Example Program to Reverse a Number
Program – reverse_number.go
</>
Copy
package main
import "fmt"
func main() {
// Declare a variable to hold the input number
var num int
// Prompt the user to enter a number
fmt.Print("Enter an integer: ")
fmt.Scan(&num)
// Initialize a variable to store the reversed number
reversed := 0
// Store the original number for display
original := num
// Reverse the number
for num != 0 {
digit := num % 10 // Extract the last digit
reversed = reversed*10 + digit // Build the reversed number
num /= 10 // Remove the last digit
}
// Display the reversed number
fmt.Printf("The reverse of %d is %d\n", original, reversed)
}
Explanation of Program
- Declare Variables: Two integer variables are declared.
num
stores the user input, andreversed
is initialized to 0 to store the reversed number. - Prompt the User: The program prompts the user to enter an integer using the
fmt.Print
function. - Extract and Reverse Digits: A loop is used to extract each digit of the number using the modulus operator (
%
). The digits are added toreversed
, multiplied by 10 to shift the digits left. - Remove Last Digit: The number is divided by 10 in each iteration to remove the last digit.
- Display the Result: The reversed number is displayed using
fmt.Printf
, along with the original number for reference.
Output
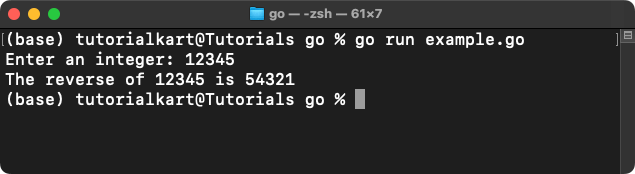
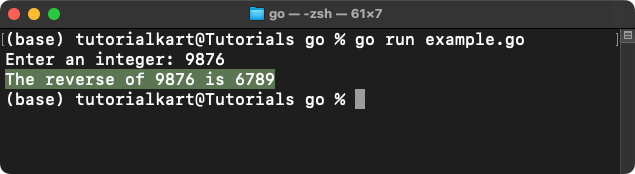
This program successfully reverses the digits of a number by iteratively extracting and rearranging its digits.