Go – Slice Append
In Go, slices are dynamic and can grow or shrink in size. The append
function is a built-in feature that allows you to add elements to the end of a slice. This makes slices highly versatile and suitable for dynamic collections where the size of the data is not fixed.
In this tutorial, we will learn how to use the append
function with slices, including appending single elements, multiple elements, and slices to other slices. Practical examples and explanations are provided to help you understand the concept.
Syntax for Append Function
The syntax of the append
function is:
slice = append(slice, elements...)
Here:
slice
: The existing slice to which elements are appended.elements
: The elements to be appended to the slice. This can include one or more elements or another slice.... (variadic operator)
: Allows appending multiple elements or another slice.
Examples of Slice Append
1. Append a Single Element
This example demonstrates how to append a single element to a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Append a single element
numbers = append(numbers, 40)
// Print the updated slice
fmt.Println("Updated Slice:", numbers)
}
Explanation
- Declare Slice: The slice
numbers
is initialized with elements{10, 20, 30}
. - Append Element: The element
40
is appended to the slice using theappend
function. - Update Slice: The
append
function returns a new slice, which replaces the original slice.
Output
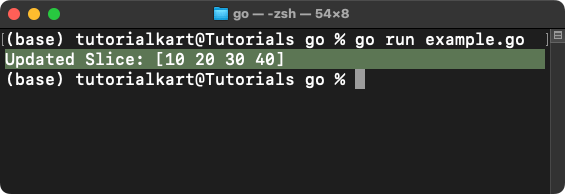
2. Append Multiple Elements
This example demonstrates how to append multiple elements to a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Append multiple elements
numbers = append(numbers, 40, 50, 60)
// Print the updated slice
fmt.Println("Updated Slice:", numbers)
}
Explanation
- Declare Slice: The slice
numbers
is initialized with elements{10, 20, 30}
. - Append Elements: Multiple elements
{40, 50, 60}
are appended using theappend
function. - Update Slice: The new slice with the additional elements replaces the original slice.
Output
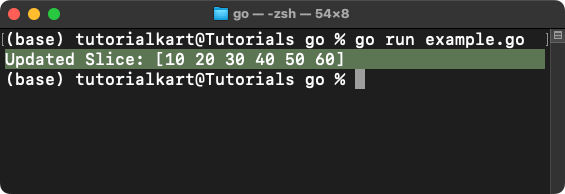
3. Append a Slice to Another Slice
This example demonstrates how to append all elements of one slice to another slice:
package main
import "fmt"
func main() {
// Declare and initialize two slices
slice1 := []int{1, 2, 3}
slice2 := []int{4, 5, 6}
// Append slice2 to slice1
slice1 = append(slice1, slice2...)
// Print the updated slice
fmt.Println("Updated Slice:", slice1)
}
Explanation
- Declare Slices: Two slices
slice1
andslice2
are declared and initialized. - Append Slice: The elements of
slice2
are appended toslice1
usingappend(slice1, slice2...)
. - Update Slice: The resulting slice is stored in
slice1
.
Output
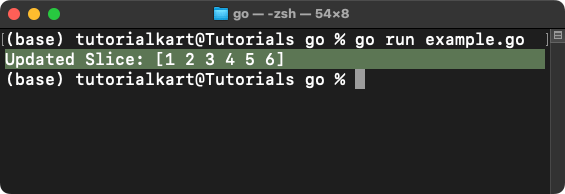
Points to Remember
- Dynamic Growth: Slices can grow dynamically using the
append
function. - New Slice: The
append
function returns a new slice, which may have a different underlying array if the capacity of the original slice is exceeded. - Variadic Operator: Use
...
to append all elements of one slice to another. - Efficiency: Appending to slices is efficient, but frequent resizing of the underlying array may impact performance for large datasets.