Go Slice Best Practices
Slices in Go are a powerful and versatile data structure that provides a dynamic and efficient way to manage collections of elements. However, improper use of slices can lead to performance issues, unexpected behavior, or inefficient memory usage. By following best practices, you can ensure that your slice operations are efficient, safe, and maintainable.
In this tutorial, we will explore best practices for working with slices in Go, supported by examples and explanations to help you write better slice-related code.
Best Practices for Using Slices in Go
- Use
make()
for Slice Initialization: Initialize slices usingmake
with an appropriate length and capacity to optimize memory usage. - Avoid Modifying Shared Data: Be cautious when multiple slices share the same underlying array to prevent unintended side effects.
- Leverage
append()
: Useappend()
for dynamic growth instead of manually resizing slices. - Use
copy()
for Independence: When slices need to be independent, usecopy()
to duplicate data. - Manage Capacity Growth: Preallocate capacity when possible to reduce the overhead of dynamic resizing.
- Check Bounds: Always validate indices to avoid runtime errors like
index out of range
. - Clear Slices Properly: Use re-slicing or assignment for clearing slices instead of manually deleting elements.
- Understand Shared References: Be aware that slicing creates a reference to the same underlying array and does not copy data.
Examples of Best Practices with Slices
1 Initialize Slices with make()
Using make()
ensures slices are initialized with the desired length and capacity for efficient memory usage:
package main
import "fmt"
func main() {
// Initialize a slice with make
slice := make([]int, 5, 10)
// Print the length and capacity
fmt.Println("Length:", len(slice))
fmt.Println("Capacity:", cap(slice))
}
Explanation
- Define Length and Capacity: The slice is initialized with a length of 5 and a capacity of 10.
- Efficient Memory Allocation: This approach preallocates memory, reducing the need for dynamic resizing during growth.
Output
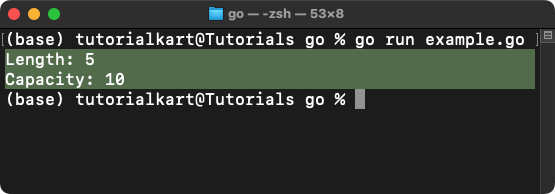
2 Avoid Modifying Shared Data
Multiple slices sharing the same array can lead to unintended side effects:
package main
import "fmt"
func main() {
// Create an array and two slices sharing it
arr := [5]int{10, 20, 30, 40, 50}
slice1 := arr[1:4]
slice2 := arr[2:5]
// Modify slice1
slice1[1] = 99
// Print the array and slices
fmt.Println("Array:", arr)
fmt.Println("Slice1:", slice1)
fmt.Println("Slice2:", slice2)
}
Explanation
- Shared Data: Both slices reference the same underlying array.
- Unintended Modification: Changing
slice1
affects both the array andslice2
.
Output
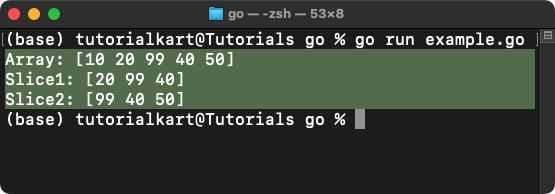
3 Use copy()
for Independent Slices
To avoid shared data issues, use copy()
to create independent slices:
package main
import "fmt"
func main() {
// Original slice
original := []int{1, 2, 3, 4, 5}
// Create an independent copy
copySlice := make([]int, len(original))
copy(copySlice, original)
// Modify the copied slice
copySlice[0] = 99
// Print the slices
fmt.Println("Original Slice:", original)
fmt.Println("Copied Slice:", copySlice)
}
Explanation
- Create Independent Slice: A new slice
copySlice
is created withmake()
. - Copy Elements: The
copy()
function duplicates elements from the original slice. - Independent Modification: Changes to
copySlice
do not affect the original slice.
Output
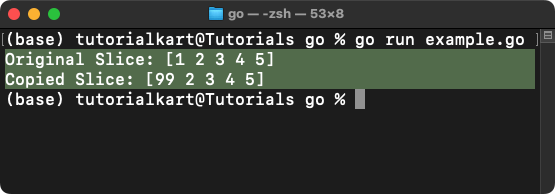
Points to Remember
- Efficient Memory Management: Preallocate slice capacity when possible to reduce dynamic resizing.
- Shared References: Understand that slices share their underlying array unless explicitly copied.
- Dynamic Growth: Use
append()
for adding elements dynamically to slices. - Bounds Checking: Always validate indices to avoid runtime errors.