Go – Slice Bounds Out of Range
In Go, slices are dynamic collections that allow you to access and manipulate data efficiently. However, accessing a slice using indices outside its valid range will result in a runtime error: panic: runtime error: slice bounds out of range
. Understanding and preventing this error is essential when working with slices.
In this tutorial, we will explore what causes the “slice bounds out of range” error, provide examples of common scenarios that lead to it, and discuss how to prevent and handle it effectively.
What Causes Slice Bounds Out of Range Error?
The “slice bounds out of range” error occurs when you attempt to access elements of a slice using an index or range that exceeds its capacity or is less than zero. This includes:
- Accessing an element with a negative index.
- Accessing an element at an index equal to or greater than the slice length.
- Creating a subslice with indices that exceed the slice boundaries.
Examples of Slice Bounds Out of Range
1. Accessing an Element Outside the Range
This example demonstrates how accessing an element outside the range of a slice leads to an error:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Try to access an element out of range
fmt.Println(numbers[3]) // This will cause a panic
}
Output
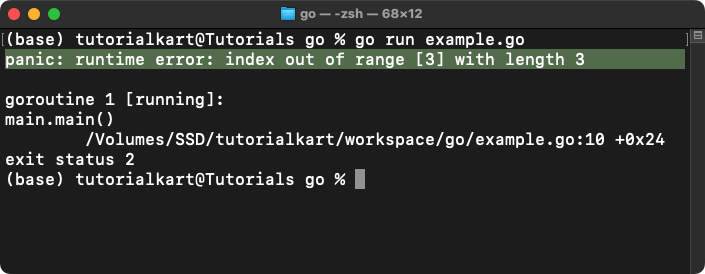
2. Creating a Subslice with Invalid Indices
This example demonstrates how using indices outside the valid range when creating a subslice leads to an error:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Try to create a subslice with invalid indices
fmt.Println(numbers[1:4]) // This will cause a panic
}
Output
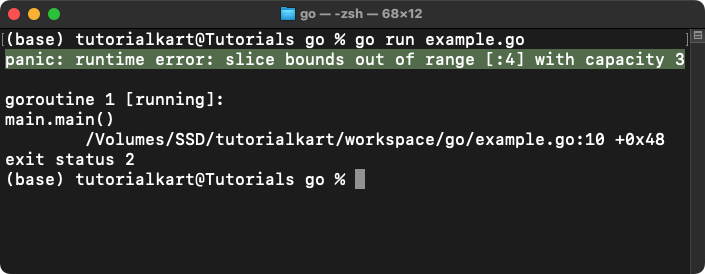
How to Prevent Slice Bounds Out of Range Error
- Check Slice Length: Always check the length of the slice using
len(slice)
before accessing elements or creating subslices. - Use Conditional Statements: Validate indices with conditional checks to ensure they are within the valid range.
- Handle Empty Slices: Ensure the slice is not empty before accessing its elements.
Example of Preventing Slice Bounds Out of Range
Safe Access with Index Validation
This example demonstrates how to safely access a slice element using index validation:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Validate index before accessing the element
index := 3
if index >= 0 && index < len(numbers) {
fmt.Println("Element at index", index, ":", numbers[index])
} else {
fmt.Println("Index out of range:", index)
}
}
Output
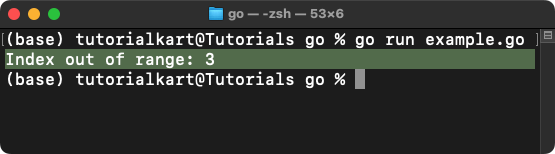
Points to Remember
- Always Validate Indices: Ensure that indices are within the valid range of the slice before accessing elements or creating subslices.
- Check Slice Length: Use
len(slice)
to get the length of a slice and ensure your operations are safe. - Handle Edge Cases: Consider scenarios where the slice may be empty or shorter than expected.
- Use Defensive Programming: Implement checks and error handling to make your code robust against runtime errors.