Go – Slice Capacity
In Go, slices are dynamic and can grow or shrink in size. While slices have a length
that represents the number of elements they contain, they also have a capacity
, which is the maximum number of elements the slice can hold without reallocating its underlying array. Understanding slice capacity is important for optimizing performance and managing memory efficiently.
In this tutorial, we will explore how to determine and use slice capacity in Go, along with practical examples and explanations.
Syntax for Slice Capacity
You can find the capacity of a slice using the cap
function:
capacity := cap(slice)
Here:
slice
: The slice whose capacity you want to determine.capacity
: The maximum number of elements the slice can hold.
Examples of Slice Capacity
1. Determine Capacity of a Slice
This example demonstrates how to find the capacity of a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Print the capacity of the slice
fmt.Println("Capacity of Slice:", cap(numbers))
}
Explanation
- Declare Slice: The slice
numbers
is initialized with elements{10, 20, 30, 40, 50}
. - Determine Capacity: The
cap
function is used to find the capacity of the slice, which is equal to the number of elements in the underlying array. - Print Capacity: The capacity is printed using
fmt.Println
.
Output
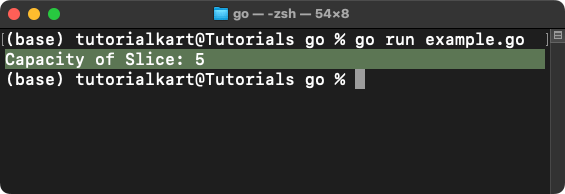
2. Capacity After Slicing
This example demonstrates how slicing affects the capacity of a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Create a new slice by slicing the original slice
subSlice := numbers[1:4]
// Print the capacity of the new slice
fmt.Println("Capacity of SubSlice:", cap(subSlice))
}
Explanation
- Declare Slice: The slice
numbers
is initialized with elements{10, 20, 30, 40, 50}
. - Create SubSlice: A new slice
subSlice
is created fromnumbers[1:4]
, which includes elements{20, 30, 40}
. - Determine Capacity: The capacity of
subSlice
includes elements from its starting index to the end of the underlying array.
Output
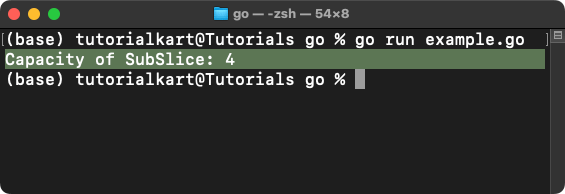
3. Capacity After Appending Elements
This example demonstrates how appending elements to a slice can change its capacity:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Print initial capacity
fmt.Println("Initial Capacity:", cap(numbers))
// Append elements to the slice
numbers = append(numbers, 40, 50, 60)
// Print updated capacity
fmt.Println("Updated Capacity:", cap(numbers))
}
Explanation
- Declare Slice: The slice
numbers
is initialized with elements{10, 20, 30}
. - Print Initial Capacity: The initial capacity is printed using
cap
. - Append Elements: Additional elements
{40, 50, 60}
are appended to the slice. If the capacity of the underlying array is exceeded, a new array is allocated with increased capacity. - Print Updated Capacity: The updated capacity is printed using
cap
.
Output
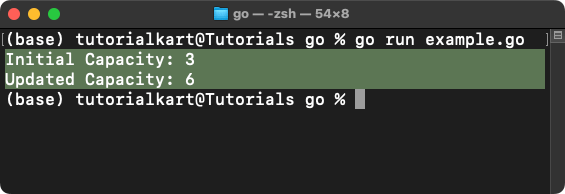
Points to Remember
- Capacity: The capacity of a slice represents the maximum number of elements it can hold before reallocating the underlying array.
- Effect of Slicing: The capacity of a slice created from another slice includes elements from its starting index to the end of the original array.
- Dynamic Growth: Appending elements to a slice may increase its capacity by allocating a new, larger array.
- Performance: Managing slice capacity efficiently can improve performance and reduce memory usage.