Go – Slice Capacity vs Length
In Go, slices are dynamic data structures with two important properties: length
and capacity
. These properties provide essential information about the slice, such as the number of elements it currently holds and the maximum number of elements it can hold without reallocating its underlying array. Understanding the difference between length and capacity is crucial for effective slice management.
In this tutorial, we will explore the differences between slice length and capacity with practical examples and explanations.
Key Differences Between Slice Length and Capacity
The following table summarizes the key differences between slice length and capacity:
Property | Description | Function |
---|---|---|
Length | The number of elements the slice currently holds. | len(slice) |
Capacity | The maximum number of elements the slice can hold without reallocating the underlying array. | cap(slice) |
Dynamic Behavior | Changes when elements are added or removed using slicing or appending. | Dynamic adjustment via slicing or append operations. |
Underlying Array | Length is always ≤ capacity. | N/A |
Examples of Slice Length and Capacity
1. Initial Slice Length and Capacity
This example demonstrates how to determine the initial length and capacity of a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Print the length and capacity
fmt.Println("Length of Slice:", len(numbers))
fmt.Println("Capacity of Slice:", cap(numbers))
}
Explanation
- Declare Slice: The slice
numbers
is initialized with elements{10, 20, 30, 40, 50}
. - Length: The length of the slice is
5
, which is the number of elements it holds. - Capacity: The capacity of the slice is also
5
, as it is created with a backing array of the same size.
Output
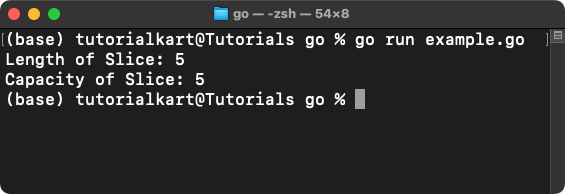
2. Length and Capacity After Slicing
This example demonstrates how slicing affects the length and capacity of a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Create a new slice by slicing the original slice
subSlice := numbers[1:4]
// Print the length and capacity of the new slice
fmt.Println("Length of SubSlice:", len(subSlice))
fmt.Println("Capacity of SubSlice:", cap(subSlice))
}
Explanation
- Create SubSlice: A new slice
subSlice
is created fromnumbers[1:4]
, which includes elements{20, 30, 40}
. - Length: The length of
subSlice
is3
, as it holds three elements. - Capacity: The capacity of
subSlice
is4
, as it includes elements from the start index to the end of the original slice’s backing array.
Output
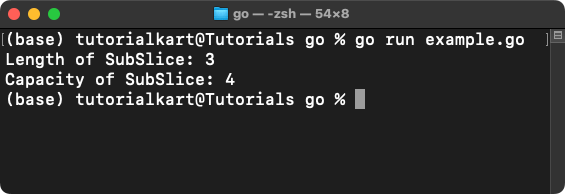
3. Length and Capacity After Appending Elements
This example demonstrates how appending elements affects the length and capacity of a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Print initial length and capacity
fmt.Println("Initial Length:", len(numbers))
fmt.Println("Initial Capacity:", cap(numbers))
// Append elements to the slice
numbers = append(numbers, 40, 50, 60)
// Print updated length and capacity
fmt.Println("Updated Length:", len(numbers))
fmt.Println("Updated Capacity:", cap(numbers))
}
Explanation
- Initial State: The slice
numbers
is initialized with elements{10, 20, 30}
, having a length of3
and capacity of3
. - Append Elements: Additional elements
{40, 50, 60}
are appended. If the capacity is exceeded, a new underlying array is allocated with increased capacity. - Updated State: The length increases to
6
, and the capacity is updated to accommodate the new elements.
Output
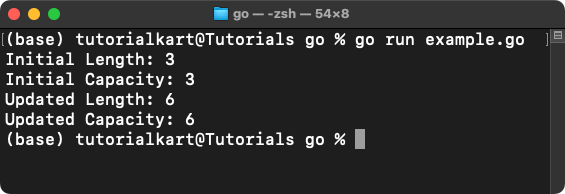
Points to Remember
- Length: The number of elements currently in the slice.
- Capacity: The maximum number of elements the slice can hold without reallocating the underlying array.
- Slicing: Slicing a slice reduces its length but may retain the full capacity of the original slice.
- Appending: Adding elements to a slice can increase its length, and if the capacity is exceeded, the slice is reallocated with a larger underlying array.