Go Slice Clear
In Go, clearing a slice means removing all its elements or resetting its length to zero. While Go does not have a built-in method like clear()
in some other languages, you can achieve this by re-slicing or assigning a new slice. Clearing a slice does not affect its underlying array unless explicitly handled.
In this tutorial, we will explore how to clear a slice in Go using various methods with examples and detailed explanations.
Methods to Clear a Slice
- Re-slicing: Set the slice length to zero while retaining the underlying array.
- Assigning a New Slice: Assign an empty slice to the variable, effectively replacing the original slice.
- Clearing Underlying Data: Explicitly set all elements in the underlying array to their default values.
Examples of Clearing a Slice
1. Clear a Slice Using Re-Slicing
This example demonstrates how to clear a slice by re-slicing it to zero length:
</>
Copy
package main
import "fmt"
func main() {
// Initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Clear the slice by re-slicing
numbers = numbers[:0]
// Print the result
fmt.Println("Cleared Slice:", numbers)
fmt.Println("Length:", len(numbers))
fmt.Println("Capacity:", cap(numbers))
}
Explanation
- Initialize Slice: A slice named
numbers
is initialized with five elements. - Re-slicing: The slice is re-sliced using
numbers[:0]
, setting its length to zero while retaining its capacity and underlying array. - Print Results: The cleared slice is printed, showing an empty slice with a length of
0
but the same capacity as the original slice.
Output
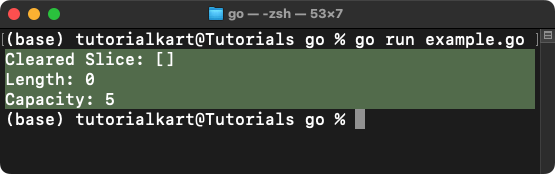
2. Clear a Slice by Assigning a New Slice
This example demonstrates how to clear a slice by assigning a new, empty slice:
</>
Copy
package main
import "fmt"
func main() {
// Initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Clear the slice by assigning a new slice
numbers = []int{}
// Print the result
fmt.Println("Cleared Slice:", numbers)
fmt.Println("Length:", len(numbers))
fmt.Println("Capacity:", cap(numbers))
}
Explanation
- Initialize Slice: A slice named
numbers
is initialized with five elements. - Assign a New Slice: A new empty slice (
[]int{}
) is assigned to thenumbers
variable, effectively clearing it. - Print Results: The cleared slice is printed, showing an empty slice with both length and capacity set to
0
.
Output
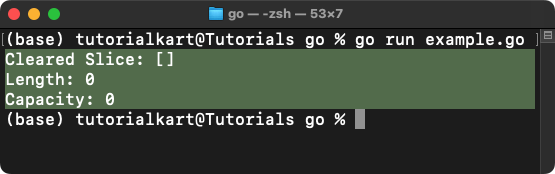
3. Clear the Underlying Data
This example demonstrates how to clear a slice by resetting all elements in the underlying array:
</>
Copy
package main
import "fmt"
func main() {
// Initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Clear the underlying data
for i := range numbers {
numbers[i] = 0
}
// Print the result
fmt.Println("Cleared Slice:", numbers)
}
Explanation
- Initialize Slice: A slice named
numbers
is initialized with five elements. - Reset Elements: A
for
loop iterates through the slice, setting each element to its default value (0
for integers). - Print Results: The cleared slice is printed, showing all elements set to
0
.
Output
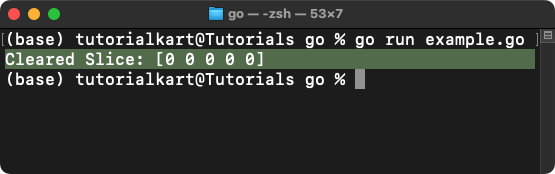
Points to Remember
- Re-Slicing: Setting the length to zero retains the slice’s capacity and underlying array.
- New Slice: Assigning a new slice resets both length and capacity to zero, releasing the reference to the original array.
- Underlying Data: Resetting the underlying array explicitly clears the data but retains the slice’s length and capacity.
- Memory Management: Be mindful of memory usage when clearing slices, especially if they retain references to large arrays.