Go – Slice Comparison
In Go, slices are dynamic data structures used to store a collection of elements. Comparing slices in Go is not straightforward because slices cannot be directly compared using comparison operators like ==
. However, you can use custom logic or built-in packages like reflect
to compare slices for equality.
In this tutorial, we will learn how to compare slices in Go using different approaches, along with practical examples and explanations.
Key Points About Slice Comparison
- Direct Comparison: You cannot directly compare slices using operators like
==
, except fornil
comparison. - Custom Logic: You can write custom functions to compare slices by iterating through their elements.
- Reflect Package: The
reflect.DeepEqual
function can be used for deep comparison of slices.
Examples of Slice Comparison
1 Compare Slices Using Custom Logic
This example demonstrates how to compare slices element by element:
</>
Copy
package main
import "fmt"
// Function to compare two slices
func areSlicesEqual(slice1, slice2 []int) bool {
if len(slice1) != len(slice2) {
return false
}
for i := range slice1 {
if slice1[i] != slice2[i] {
return false
}
}
return true
}
func main() {
// Declare and initialize two slices
slice1 := []int{1, 2, 3}
slice2 := []int{1, 2, 3}
slice3 := []int{1, 2, 4}
// Compare slices
fmt.Println("Are slice1 and slice2 equal?", areSlicesEqual(slice1, slice2)) // true
fmt.Println("Are slice1 and slice3 equal?", areSlicesEqual(slice1, slice3)) // false
}
Explanation
- Length Check: The function first checks if the lengths of the slices are equal. If not, they are not equal.
- Element Comparison: A
for
loop iterates through the slices, comparing elements at the same index. - Return Result: The function returns
true
if all elements are equal, otherwise it returnsfalse
.
Output
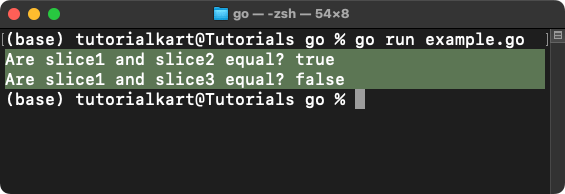
2 Compare Slices Using reflect.DeepEqual
This example demonstrates how to compare slices using the reflect.DeepEqual
function:
</>
Copy
package main
import (
"fmt"
"reflect"
)
func main() {
// Declare and initialize two slices
slice1 := []int{1, 2, 3}
slice2 := []int{1, 2, 3}
slice3 := []int{1, 2, 4}
// Compare slices using reflect.DeepEqual
fmt.Println("Are slice1 and slice2 equal?", reflect.DeepEqual(slice1, slice2)) // true
fmt.Println("Are slice1 and slice3 equal?", reflect.DeepEqual(slice1, slice3)) // false
}
Explanation
- Import reflect: The
reflect
package provides theDeepEqual
function for deep comparison of slices. - Compare Slices:
DeepEqual
compares the elements and structure of the slices. - Return Result: The function returns
true
if the slices are equal, otherwise it returnsfalse
.
Output
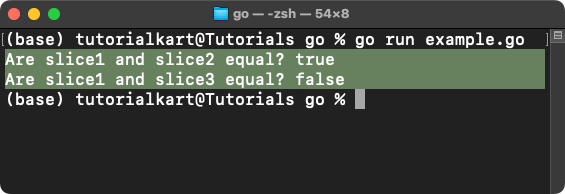
3 Compare Slices for nil
You can directly compare slices with nil
to check if a slice is uninitialized:
</>
Copy
package main
import "fmt"
func main() {
var slice1 []int // nil slice
slice2 := []int{} // empty slice
fmt.Println("Is slice1 nil?", slice1 == nil) // true
fmt.Println("Is slice2 nil?", slice2 == nil) // false
}
Explanation
- Nil Slice: A nil slice is uninitialized and points to
nil
. - Empty Slice: An empty slice is initialized but contains no elements. It is not
nil
. - Direct Comparison: You can directly compare slices with
nil
to determine their initialization state.
Output
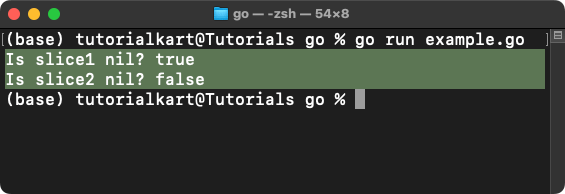
Points to Remember
- Direct Comparison: Slices cannot be directly compared for equality except with
nil
. - Custom Logic: Write a function to compare slices element by element for manual control.
- reflect.DeepEqual: Use
reflect.DeepEqual
for convenient and accurate deep comparison of slices. - Performance: Custom comparison may be faster than
reflect.DeepEqual
for large slices in performance-critical applications.