Go – Slice Concat
In Go, slices are dynamic and allow flexible operations, including concatenation. Concatenating slices involves combining the elements of two or more slices into a single slice. This operation can be performed using the built-in append
function and the variadic operator (...
).
In this tutorial, we will learn how to concatenate slices in Go, covering different scenarios with practical examples and detailed explanations.
Syntax for Slice Concatenation
The syntax for concatenating slices is:
</>
Copy
resultSlice := append(slice1, slice2...)
Here:
slice1
: The first slice to which elements are added.slice2
: The second slice whose elements are concatenated toslice1
.resultSlice
: The resulting slice containing all elements ofslice1
followed by all elements ofslice2
....
: The variadic operator, which expandsslice2
into individual elements for appending.
Examples of Slice Concatenation
1 Concatenate Two Slices
This example demonstrates how to concatenate two slices:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize two slices
slice1 := []int{1, 2, 3}
slice2 := []int{4, 5, 6}
// Concatenate the slices
result := append(slice1, slice2...)
// Print the resulting slice
fmt.Println("Concatenated Slice:", result)
}
Explanation
- Declare Slices: Two slices
slice1
andslice2
are initialized with values{1, 2, 3}
and{4, 5, 6}
, respectively. - Concatenate Slices: The elements of
slice2
are appended toslice1
using theappend
function with the variadic operator (...
). - Print Result: The resulting slice is printed using
fmt.Println
.
Output
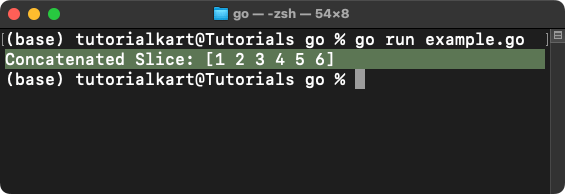
2 Concatenate Multiple Slices
This example demonstrates how to concatenate multiple slices into a single slice:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize three slices
slice1 := []int{1, 2}
slice2 := []int{3, 4}
slice3 := []int{5, 6}
// Concatenate the slices
result := append(slice1, append(slice2, slice3...)...)
// Print the resulting slice
fmt.Println("Concatenated Slice:", result)
}
Explanation
- Declare Slices: Three slices
slice1
,slice2
, andslice3
are initialized with values{1, 2}
,{3, 4}
, and{5, 6}
, respectively. - Concatenate Slices:
slice2
andslice3
are concatenated first, and their result is concatenated withslice1
. - Print Result: The resulting slice is printed using
fmt.Println
.
Output
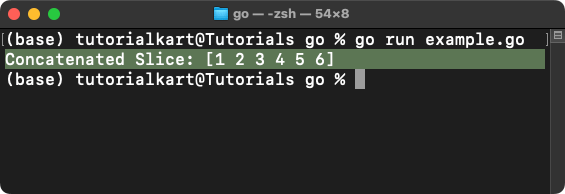
3 Concatenate Slices of Strings
This example demonstrates how to concatenate slices of strings:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize two slices of strings
slice1 := []string{"Hello", "World"}
slice2 := []string{"from", "Go"}
// Concatenate the slices
result := append(slice1, slice2...)
// Print the resulting slice
fmt.Println("Concatenated Slice:", result)
}
Explanation
- Declare Slices: Two slices of strings
slice1
andslice2
are initialized with values{"Hello", "World"}
and{"from", "Go"}
, respectively. - Concatenate Slices: The elements of
slice2
are appended toslice1
using theappend
function with the variadic operator (...
). - Print Result: The resulting slice is printed using
fmt.Println
.
Output
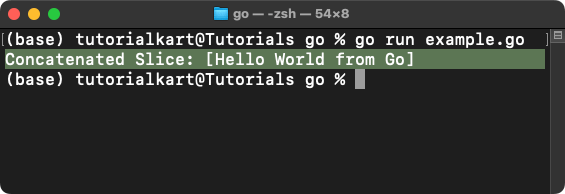
Points to Remember
- Variadic Operator: The variadic operator (
...
) is required to expand a slice into individual elements when usingappend
. - Dynamic Growth: Concatenating slices creates a new slice that can accommodate all the elements, possibly reallocating memory if necessary.
- Type Consistency: All slices being concatenated must have the same element type.
- Nested Concatenation: Multiple slices can be concatenated in a single operation by nesting
append
calls.