Go Slice Copy
In Go, slices are references to an underlying array, meaning modifications to one slice can affect other slices referencing the same data. To create a separate copy of a slice with its own underlying array, you can use the built-in copy()
function.
In this tutorial, we will explore how to use the copy()
function to duplicate slices, examine its behavior with different lengths, and provide practical examples with detailed explanations.
Steps to Copy a Slice
- Create the Destination Slice: Allocate memory for the destination slice with sufficient length.
- Use the
copy()
Function: Callcopy(dest, src)
to copy elements from the source slice to the destination slice. - Handle Length Mismatch: If the destination slice is shorter than the source, only the overlapping elements will be copied.
Examples of Slice Copy
1 Copy a Slice with Equal Length
This example demonstrates how to copy a slice when the source and destination slices have equal lengths:
package main
import "fmt"
func main() {
// Source slice
source := []int{10, 20, 30, 40, 50}
// Destination slice with the same length
destination := make([]int, len(source))
// Copy the source slice to the destination slice
copy(destination, source)
// Print the source and destination slices
fmt.Println("Source Slice:", source)
fmt.Println("Destination Slice:", destination)
}
Explanation
- Create Source Slice: The
source
slice is initialized with five integers. - Create Destination Slice: The
destination
slice is created with the same length as thesource
slice usingmake([]int, len(source))
. - Copy Elements: The
copy(destination, source)
function copies all elements fromsource
todestination
. - Print Results: Both slices are printed to confirm the successful copy.
Output
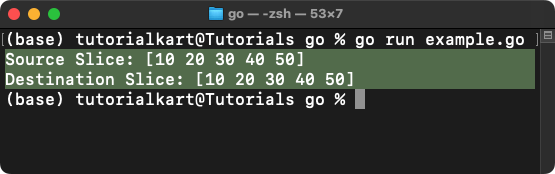
2 Copy a Slice with Shorter Destination
This example demonstrates how to copy a slice when the destination slice is shorter than the source slice:
package main
import "fmt"
func main() {
// Source slice
source := []int{10, 20, 30, 40, 50}
// Destination slice with a shorter length
destination := make([]int, 3)
// Copy the source slice to the destination slice
copy(destination, source)
// Print the source and destination slices
fmt.Println("Source Slice:", source)
fmt.Println("Destination Slice:", destination)
}
Explanation
- Create Source Slice: The
source
slice is initialized with five integers. - Create Destination Slice: The
destination
slice is created with a shorter length of3
usingmake([]int, 3)
. - Copy Overlapping Elements: The
copy(destination, source)
function copies only the first three elements fromsource
todestination
, as the destination slice cannot accommodate more. - Print Results: The slices are printed to show that only the overlapping elements were copied.
Output
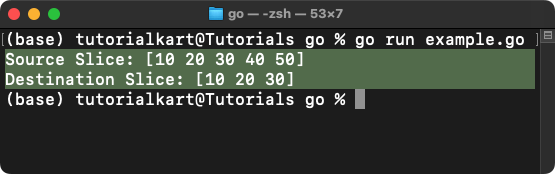
3 Copy a Slice to a Larger Destination
This example demonstrates how to copy a slice when the destination slice is larger than the source slice:
package main
import "fmt"
func main() {
// Source slice
source := []int{10, 20, 30}
// Destination slice with a larger length
destination := make([]int, 5)
// Copy the source slice to the destination slice
copy(destination, source)
// Print the source and destination slices
fmt.Println("Source Slice:", source)
fmt.Println("Destination Slice:", destination)
}
Explanation
- Create Source Slice: The
source
slice is initialized with three integers. - Create Destination Slice: The
destination
slice is created with a larger length of5
usingmake([]int, 5)
. - Copy Elements: The
copy(destination, source)
function copies all elements fromsource
to the first three positions indestination
, leaving the remaining positions as their default value (0
). - Print Results: The slices are printed to show that the source slice elements were copied and additional space remains in the destination slice.
Output
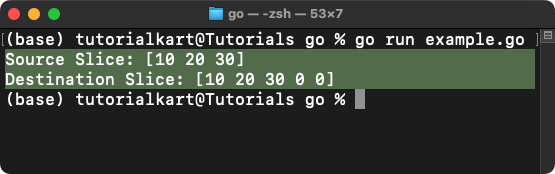
Points to Remember
- Efficient Copying: The
copy()
function is optimized for copying slices efficiently. - Length Mismatch: When the destination slice is shorter than the source, only the overlapping elements are copied.
- Mutual Independence: After copying, the destination slice is independent of the source slice.
- Default Values: If the destination slice is longer than the source slice, extra positions are initialized with default values of the element type.