Go – Slice Count Occurrences
In Go, slices are dynamic data structures that allow flexible operations, including counting occurrences of specific elements. While Go does not have a built-in function to directly count elements in a slice, you can implement custom logic to achieve this using loops and conditional statements.
In this tutorial, we will learn how to count the occurrences of specific elements in a slice with practical examples and explanations.
Counting Elements in a Slice
To count the occurrences of an element in a slice, you can iterate through the slice using a for
loop and increment a counter each time the element matches your target value.
func countOccurrences(slice []int, target int) int {
count := 0
for _, v := range slice {
if v == target {
count++
}
}
return count
}
This function takes a slice and a target value as input and returns the number of occurrences of the target value in the slice.
Examples of Counting Elements in a Slice
1. Count Occurrences of an Integer
This example demonstrates how to count occurrences of an integer in a slice:
package main
import "fmt"
// Function to count occurrences of a target integer in a slice
func countOccurrences(slice []int, target int) int {
count := 0
for _, v := range slice {
if v == target {
count++
}
}
return count
}
func main() {
// Declare and initialize a slice
numbers := []int{1, 2, 3, 2, 4, 2, 5}
// Count occurrences of the number 2
result := countOccurrences(numbers, 2)
// Print the result
fmt.Println("Occurrences of 2:", result)
}
Explanation
- Define Function: The
countOccurrences
function iterates through the slice and increments a counter whenever an element matches the target. - Call Function: The slice
numbers
and the target value2
are passed to the function. - Print Result: The number of occurrences of
2
in the slice is printed usingfmt.Println
.
Output
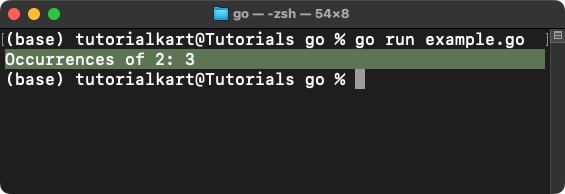
2. Count Occurrences of a String
This example demonstrates how to count occurrences of a string in a slice of strings:
package main
import "fmt"
// Function to count occurrences of a target string in a slice
func countOccurrences(slice []string, target string) int {
count := 0
for _, v := range slice {
if v == target {
count++
}
}
return count
}
func main() {
// Declare and initialize a slice of strings
fruits := []string{"apple", "banana", "apple", "cherry", "apple"}
// Count occurrences of "apple"
result := countOccurrences(fruits, "apple")
// Print the result
fmt.Println("Occurrences of 'apple':", result)
}
Explanation
- Define Function: The
countOccurrences
function iterates through the slice and increments a counter whenever an element matches the target string. - Call Function: The slice
fruits
and the target string"apple"
are passed to the function. - Print Result: The number of occurrences of
"apple"
in the slice is printed usingfmt.Println
.
Output
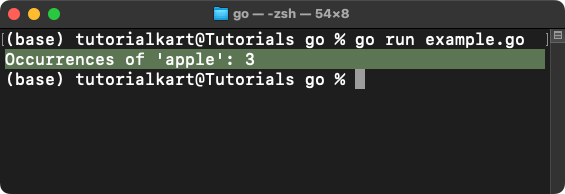
3. Count Elements Meeting a Condition
This example demonstrates how to count elements in a slice that satisfy a condition:
package main
import "fmt"
// Function to count elements greater than a given value
func countGreaterThan(slice []int, threshold int) int {
count := 0
for _, v := range slice {
if v > threshold {
count++
}
}
return count
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 15, 25, 5}
// Count elements greater than 20
result := countGreaterThan(numbers, 20)
// Print the result
fmt.Println("Elements greater than 20:", result)
}
Explanation
- Define Function: The
countGreaterThan
function iterates through the slice and increments a counter for elements greater than the threshold. - Call Function: The slice
numbers
and the threshold20
are passed to the function. - Print Result: The count of elements greater than
20
is printed usingfmt.Println
.
Output
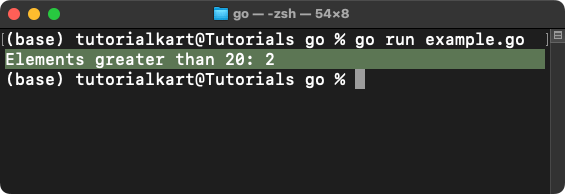
Points to Remember
- Custom Logic: Go does not have a built-in function to count elements in a slice, so you need to implement custom logic using loops.
- Generic Patterns: You can generalize counting functions to work with any type or condition using interfaces and function closures.
- Dynamic Slices: Slices in Go are dynamic, so counts may change as elements are added or removed.