Go – Slice Deep Copy
In Go, slices are references to underlying arrays, meaning changes to one slice can affect another slice that shares the same underlying array. To avoid this, you can perform a deep copy, where the data of one slice is copied to a new, independent slice. This ensures modifications to one slice do not affect the other.
In this tutorial, we will explore how to perform a deep copy of slices in Go with practical examples and detailed explanations.
Steps to Perform a Deep Copy
- Create a New Slice: Create a new slice with the same length as the original slice.
- Copy Elements: Use the
copy
function or afor
loop to copy elements from the original slice to the new slice. - Independent Memory: The new slice will have its own backing array, making it independent of the original slice.
Examples of Deep Copy in Go
1 Deep Copy Using the copy Function
This example demonstrates how to use the built-in copy
function to perform a deep copy of a slice:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
original := []int{1, 2, 3, 4, 5}
// Create a new slice with the same length as the original
copied := make([]int, len(original))
// Perform a deep copy
copy(copied, original)
// Modify the original slice
original[0] = 10
// Print both slices
fmt.Println("Original Slice:", original)
fmt.Println("Copied Slice:", copied)
}
Explanation
- Declare Original Slice: The slice
original
is initialized with values{1, 2, 3, 4, 5}
. - Create Copied Slice: The slice
copied
is created usingmake
with the same length as the original slice. - Copy Elements: The
copy
function is used to copy elements fromoriginal
tocopied
. - Modify Original: Modifying the
original
slice does not affect thecopied
slice.
Output
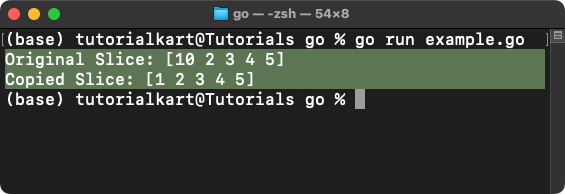
2 Deep Copy Using a for Loop
This example demonstrates how to manually copy elements using a for
loop:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
original := []string{"apple", "banana", "cherry"}
// Create a new slice with the same length as the original
copied := make([]string, len(original))
// Perform a deep copy using a for loop
for i, v := range original {
copied[i] = v
}
// Modify the original slice
original[0] = "grape"
// Print both slices
fmt.Println("Original Slice:", original)
fmt.Println("Copied Slice:", copied)
}
Explanation
- Declare Original Slice: The slice
original
is initialized with values{"apple", "banana", "cherry"}
. - Create Copied Slice: The slice
copied
is created usingmake
with the same length as the original slice. - Use for Loop: A
for
loop iterates throughoriginal
and copies each element tocopied
. - Modify Original: Changes to
original
do not affectcopied
.
Output
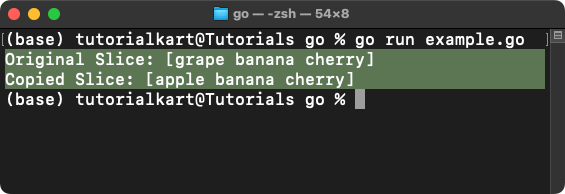
Points to Remember
- Independent Slices: A deep copy creates a new slice with its own backing array, independent of the original slice.
- Use copy Function: The
copy
function is the most efficient way to perform a deep copy in Go. - Use for Loop: A
for
loop can be used for more control over the copying process. - Memory Management: Ensure the new slice has enough capacity to hold all elements of the original slice.