Go Slice Default Value
In Go, slices are dynamic collections of elements of a specific type. When you create a slice of a particular type, its elements are initialized with the default value of that type. Understanding these default values is crucial when working with slices to avoid unexpected behavior in your programs.
In this tutorial, we will explore the default values for slices of various types, provide examples of their initialization, and discuss how to work with these default values effectively.
Default Values in Slices
- Numeric Types: The default value is
0
for integers and floating-point numbers. - String Type: The default value is an empty string (
""
). - Boolean Type: The default value is
false
. - Pointers and Interfaces: The default value is
nil
. - Structs: Each field of the struct is initialized with its respective default value.
Examples of Slice Default Values
1 Default Value in a Slice of Integers
This example demonstrates the default value of elements in a slice of integers:
package main
import "fmt"
func main() {
// Create a slice of integers with length 5
numbers := make([]int, 5)
// Print the slice
fmt.Println("Slice of Integers:", numbers)
}
Output
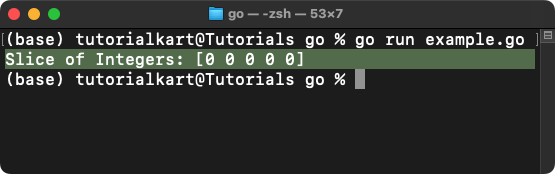
2 Default Value in a Slice of Strings
This example demonstrates the default value of elements in a slice of strings:
package main
import "fmt"
func main() {
// Create a slice of strings with length 3
words := make([]string, 3)
// Print the slice
fmt.Println("Slice of Strings:", words)
}
Output
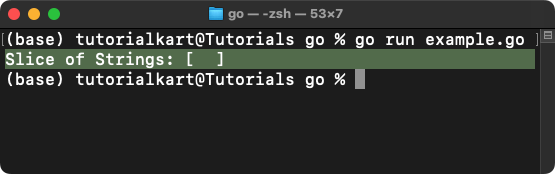
3 Default Value in a Slice of Booleans
This example demonstrates the default value of elements in a slice of booleans:
package main
import "fmt"
func main() {
// Create a slice of booleans with length 4
flags := make([]bool, 4)
// Print the slice
fmt.Println("Slice of Booleans:", flags)
}
Output
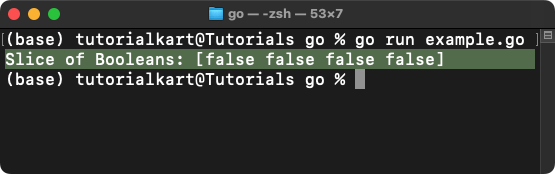
4 Default Value in a Slice of Structs
This example demonstrates the default value of elements in a slice of structs:
package main
import "fmt"
// Define a struct
type Point struct {
X int
Y int
}
func main() {
// Create a slice of structs with length 2
points := make([]Point, 2)
// Print the slice
fmt.Println("Slice of Structs:", points)
}
Output
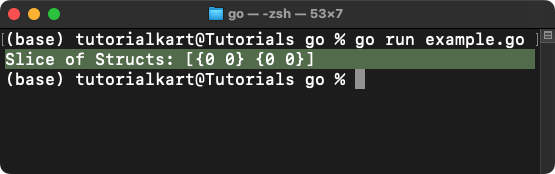
Points to Remember
- Default Values: Elements in a slice are initialized with the default value of their type.
- Using
make
: Themake
function allows you to create slices with a specified length, initializing all elements with default values. - Mutable Elements: You can modify slice elements after creation as they are initialized but not immutable.
- Custom Initialization: If default values do not suit your needs, explicitly initialize elements with specific values.