Go – Slice Difference
In Go, the difference between two slices refers to the elements that are present in the first slice but not in the second. Go does not provide a built-in function to calculate the difference, but you can implement this functionality using loops and maps for efficient comparison.
In this tutorial, we will explore how to compute the difference between two slices with practical examples and detailed explanations.
Steps to Calculate the Difference Between Two Slices
- Build a Map for the Second Slice: Use a map to store elements of the second slice for quick lookup.
- Iterate Through the First Slice: Add elements to the result slice only if they are not present in the map.
- Return the Result: The resulting slice contains the elements that are unique to the first slice.
Examples of Calculating Slice Difference
1. Difference Between Two Slices of Integers
This example demonstrates how to compute the difference between two slices of integers:
</>
Copy
package main
import "fmt"
// Function to calculate the difference between two slices
func sliceDifference(slice1, slice2 []int) []int {
diff := []int{}
elements := make(map[int]bool)
// Store elements of the second slice in a map
for _, v := range slice2 {
elements[v] = true
}
// Add elements from the first slice that are not in the map
for _, v := range slice1 {
if !elements[v] {
diff = append(diff, v)
}
}
return diff
}
func main() {
// Declare and initialize two slices
slice1 := []int{10, 20, 30, 40, 50}
slice2 := []int{30, 40, 60}
// Calculate the difference
result := sliceDifference(slice1, slice2)
// Print the result
fmt.Println("Difference:", result)
}
Explanation
- Build Map: A map is created to store the elements of
slice2
for quick lookup. - Compare Elements: The function iterates through
slice1
, checking if each element is present in the map. - Add Unique Elements: Elements from
slice1
that are not found in the map are appended to the result slice. - Print Result: The resulting slice is printed, showing the elements unique to
slice1
.
Output
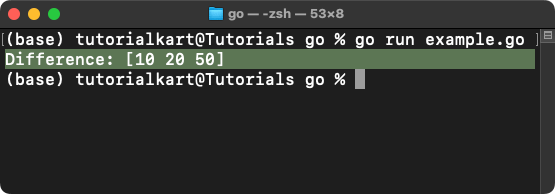
2. Difference Between Two Slices of Strings
This example demonstrates how to compute the difference between two slices of strings:
</>
Copy
package main
import "fmt"
// Function to calculate the difference between two slices
func sliceDifference(slice1, slice2 []string) []string {
diff := []string{}
elements := make(map[string]bool)
// Store elements of the second slice in a map
for _, v := range slice2 {
elements[v] = true
}
// Add elements from the first slice that are not in the map
for _, v := range slice1 {
if !elements[v] {
diff = append(diff, v)
}
}
return diff
}
func main() {
// Declare and initialize two slices
slice1 := []string{"apple", "banana", "cherry", "date"}
slice2 := []string{"banana", "cherry", "fig"}
// Calculate the difference
result := sliceDifference(slice1, slice2)
// Print the result
fmt.Println("Difference:", result)
}
Explanation
- Build Map: A map is created to store the elements of
slice2
for quick lookup. - Compare Elements: The function iterates through
slice1
, checking if each element is present in the map. - Add Unique Elements: Elements from
slice1
that are not found in the map are appended to the result slice. - Print Result: The resulting slice is printed, showing the elements unique to
slice1
.
Output
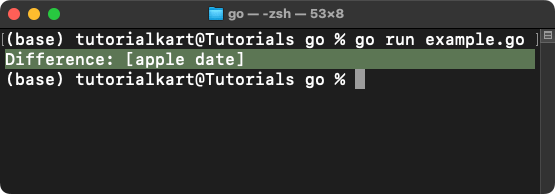
Points to Remember
- Efficiency: Using a map for lookup ensures an efficient implementation with a time complexity of
O(n+m)
, wheren
andm
are the lengths of the two slices. - Order Preservation: The order of elements in the resulting slice matches their order in the first slice.
- Generic Approach: The same logic can be applied to slices of different types, such as integers, strings, or custom structs.