Go Slice Equality
In Go, slices cannot be compared directly using the equality operator (==
), except for comparing them to nil
. To determine if two slices are equal, you need to manually compare their lengths and elements.
In this tutorial, we will explore how to check for slice equality in Go, provide examples, and explain the process in detail.
Steps to Check Slice Equality
- Compare Lengths: Check if the lengths of both slices are equal.
- Iterate Through Elements: Compare corresponding elements in both slices for equality.
- Return Result: If all elements match, the slices are equal; otherwise, they are not.
Examples of Slice Equality
1 Check Equality of Two Integer Slices
This example demonstrates how to compare two slices of integers:
</>
Copy
package main
import "fmt"
// Function to check slice equality
func slicesEqual(slice1, slice2 []int) bool {
if len(slice1) != len(slice2) {
return false
}
for i := range slice1 {
if slice1[i] != slice2[i] {
return false
}
}
return true
}
func main() {
// Declare two slices
slice1 := []int{10, 20, 30}
slice2 := []int{10, 20, 30}
// Check equality
equal := slicesEqual(slice1, slice2)
// Print the result
fmt.Println("Are slices equal?", equal)
}
Explanation
- Function Definition: A function
slicesEqual
is defined to compare the lengths and elements of two slices. - Length Check: If the lengths of the slices are different, the function returns
false
. - Element Comparison: A
for
loop iterates through the slices, comparing corresponding elements. - Return Result: If all elements match, the function returns
true
. Otherwise, it returnsfalse
.
Output
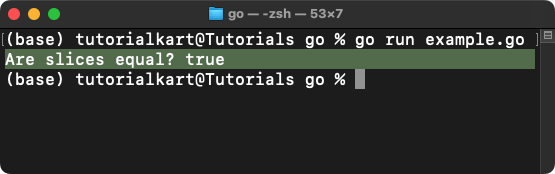
2 Compare Slices with Different Elements
This example demonstrates how the function handles slices with different elements:
</>
Copy
package main
import "fmt"
// Function to check slice equality
func slicesEqual(slice1, slice2 []int) bool {
if len(slice1) != len(slice2) {
return false
}
for i := range slice1 {
if slice1[i] != slice2[i] {
return false
}
}
return true
}
func main() {
// Declare two slices
slice1 := []int{10, 20, 30}
slice2 := []int{10, 25, 30}
// Check equality
equal := slicesEqual(slice1, slice2)
// Print the result
fmt.Println("Are slices equal?", equal)
}
Explanation
- Length Check: The lengths of both slices are equal, so the function proceeds to element comparison.
- Element Mismatch: During the comparison, the elements at index
1
differ (20
vs.25
). - Return Result: The function immediately returns
false
due to the mismatch.
Output
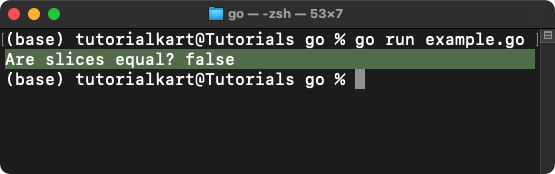
Points to Remember
- No Direct Comparison: Slices cannot be compared using
==
except fornil
checks. - Manual Comparison: Use custom functions to compare slices based on length and elements.
- Order Matters: Two slices are considered equal only if their elements match in order and value.
- Efficient Loops: A single loop is sufficient for comparing elements in most cases.