Go Slice Fill
In Go, filling a slice refers to assigning a specific value to all its elements. Since Go does not provide a built-in function to fill a slice, you can use a loop to set each element to the desired value.
In this tutorial, we will explore how to fill a slice in Go with practical examples and detailed explanations.
Steps to Fill a Slice
- Create or Initialize a Slice: Define a slice with the desired length.
- Use a Loop: Iterate through the slice and assign the desired value to each element.
- Verify the Result: Print the slice to confirm that all elements have been updated.
Examples of Filling a Slice
1. Fill a Slice of Integers
This example demonstrates how to fill a slice of integers with a specific value:
</>
Copy
package main
import "fmt"
func main() {
// Create a slice of integers
numbers := make([]int, 5)
// Fill the slice with the value 42
for i := range numbers {
numbers[i] = 42
}
// Print the filled slice
fmt.Println("Filled Slice:", numbers)
}
Explanation
- Create Slice: A slice named
numbers
is created with a length of 5 usingmake([]int, 5)
. - Iterate Through Slice: A
for
loop is used to iterate through each index of the slice. - Assign Values: Each element is assigned the value
42
. - Print Result: The slice is printed to confirm that all elements have been filled with the specified value.
Output
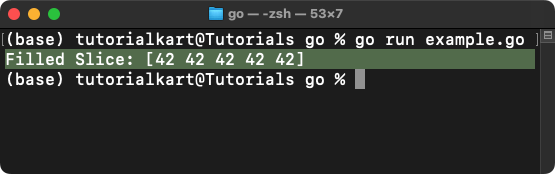
2. Fill a Slice of Strings
This example demonstrates how to fill a slice of strings with a specific value:
</>
Copy
package main
import "fmt"
func main() {
// Create a slice of strings
words := make([]string, 4)
// Fill the slice with the value "GoLang"
for i := range words {
words[i] = "GoLang"
}
// Print the filled slice
fmt.Println("Filled Slice:", words)
}
Explanation
- Create Slice: A slice named
words
is created with a length of 4 usingmake([]string, 4)
. - Iterate Through Slice: A
for
loop is used to iterate through each index of the slice. - Assign Values: Each element is assigned the value
"GoLang"
. - Print Result: The slice is printed to confirm that all elements have been filled with the specified value.
Output
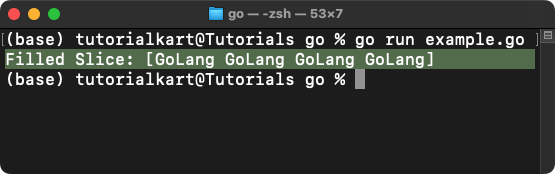
Points to Remember
- Flexible Data Types: The same approach can be used for slices of any type, such as integers, strings, or structs.
- Efficient Initialization: Filling slices is a common technique for initialization or resetting values.
- Default Values: If no explicit value is provided, slice elements are initialized with their default value (
0
for integers,false
for booleans, and""
for strings).