Go – Slice Filter
In Go, filtering a slice involves creating a new slice that contains only the elements that satisfy a specific condition. Go does not provide a built-in function for filtering slices, but you can achieve this functionality using custom functions and loops.
In this tutorial, we will explore how to filter slices in Go using practical examples and detailed explanations.
Steps to Filter a Slice
- Define a Condition: Specify a condition that determines which elements to include in the filtered slice.
- Create a New Slice: Initialize an empty slice to store the filtered elements.
- Iterate Through the Original Slice: Use a
for
loop to check each element against the condition. - Append Matching Elements: Add elements that satisfy the condition to the new slice.
Examples of Filtering Slices in Go
1 Filter Even Numbers from a Slice
This example demonstrates how to filter even numbers from a slice:
</>
Copy
package main
import "fmt"
// Function to filter even numbers
func filterEven(numbers []int) []int {
var result []int
for _, num := range numbers {
if num%2 == 0 {
result = append(result, num)
}
}
return result
}
func main() {
// Declare and initialize a slice of integers
numbers := []int{1, 2, 3, 4, 5, 6}
// Filter even numbers
evens := filterEven(numbers)
// Print the filtered slice
fmt.Println("Filtered Slice (Even Numbers):", evens)
}
Explanation
- Define Function: The
filterEven
function iterates through the slice and checks if each number is even (num%2 == 0
). - Initialize Result Slice: A new slice
result
is initialized to store the filtered elements. - Append Matching Elements: Even numbers are appended to the
result
slice usingappend
. - Return Result: The filtered slice is returned and printed in the main function.
Output
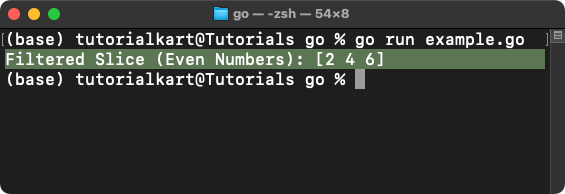
2 Filter Strings Containing a Substring
This example demonstrates how to filter strings that contain a specific substring:
</>
Copy
package main
import (
"fmt"
"strings"
)
// Function to filter strings containing a substring
func filterStrings(stringsSlice []string, substring string) []string {
var result []string
for _, str := range stringsSlice {
if strings.Contains(str, substring) {
result = append(result, str)
}
}
return result
}
func main() {
// Declare and initialize a slice of strings
words := []string{"apple", "banana", "cherry", "grape"}
// Filter strings containing "ap"
filtered := filterStrings(words, "ap")
// Print the filtered slice
fmt.Println("Filtered Slice (Contains 'ap'):", filtered)
}
Explanation
- Define Function: The
filterStrings
function iterates through the slice and checks if each string contains the specified substring usingstrings.Contains
. - Initialize Result Slice: A new slice
result
is initialized to store the matching strings. - Append Matching Elements: Strings containing the substring are appended to
result
. - Return Result: The filtered slice is returned and printed in the main function.
Output
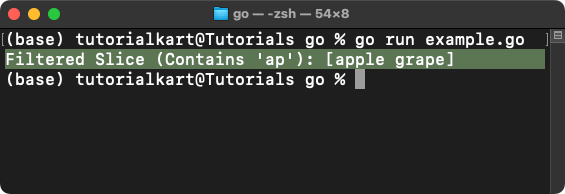
3 Filter Elements Based on Custom Logic
This example demonstrates how to use a custom filtering function to filter elements:
</>
Copy
package main
import "fmt"
// Function to filter elements based on custom logic
func filter(slice []int, condition func(int) bool) []int {
var result []int
for _, v := range slice {
if condition(v) {
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize a slice of integers
numbers := []int{1, 2, 3, 4, 5, 6}
// Filter numbers greater than 3
greaterThanThree := filter(numbers, func(n int) bool {
return n > 3
})
// Print the filtered slice
fmt.Println("Filtered Slice (Greater Than 3):", greaterThanThree)
}
Explanation
- Define Generic Function: The
filter
function takes a slice and a custom condition function as input. - Apply Condition: The condition function determines whether each element satisfies the filter criteria.
- Append Matching Elements: Elements that meet the condition are appended to the result slice.
- Return Result: The filtered slice is returned and printed in the main function.
Output
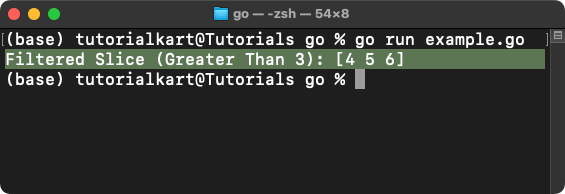
Points to Remember
- Custom Logic: Filtering requires defining a condition to determine which elements to include in the new slice.
- Dynamic Slices: The
append
function allows dynamic growth of the result slice during filtering. - Reusable Function: Generic filtering functions can be created by accepting a condition function as input.