Go – Slice Find Index
In Go, finding the index of a specific element in a slice involves iterating through the slice and checking for the target element. Since Go does not provide a built-in function to find an index, you can achieve this functionality by implementing a custom function.
In this tutorial, we will explore how to find the index of an element in a slice with practical examples and detailed explanations.
Steps to Find Index in a Slice
- Iterate Through Slice: Use a
for
loop with therange
keyword to iterate through the slice. - Compare Elements: Compare each element with the target value.
- Return Index: Return the index of the element if a match is found; otherwise, return a sentinel value like
-1
.
Examples of Finding Index in a Slice
1. Find Index of an Integer in a Slice
This example demonstrates how to find the index of an integer in a slice:
</>
Copy
package main
import "fmt"
// Function to find the index of an integer in a slice
func findIndex(slice []int, target int) int {
for i, v := range slice {
if v == target {
return i
}
}
return -1
}
func main() {
// Declare and initialize a slice of integers
numbers := []int{10, 20, 30, 40, 50}
// Find the index of 30
index := findIndex(numbers, 30)
// Print the result
fmt.Println("Index of 30:", index)
}
Explanation
- Define Function: The
findIndex
function iterates through the slice and compares each element with the target value. - Return Index: If a match is found, the function returns the index of the element; otherwise, it returns
-1
. - Call Function: The slice
numbers
and the target value30
are passed to the function, and the index is printed.
Output
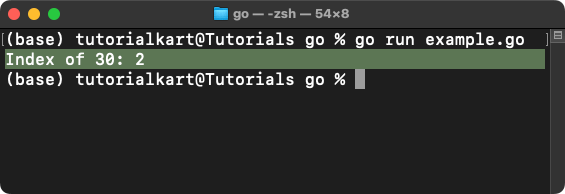
2. Find Index of a String in a Slice
This example demonstrates how to find the index of a string in a slice of strings:
</>
Copy
package main
import "fmt"
// Function to find the index of a string in a slice
func findStringIndex(slice []string, target string) int {
for i, v := range slice {
if v == target {
return i
}
}
return -1
}
func main() {
// Declare and initialize a slice of strings
fruits := []string{"apple", "banana", "cherry", "date"}
// Find the index of "cherry"
index := findStringIndex(fruits, "cherry")
// Print the result
fmt.Println("Index of 'cherry':", index)
}
Explanation
- Define Function: The
findStringIndex
function iterates through the slice and compares each string with the target value. - Return Index: If a match is found, the function returns the index of the string; otherwise, it returns
-1
. - Call Function: The slice
fruits
and the target string"cherry"
are passed to the function, and the index is printed.
Output
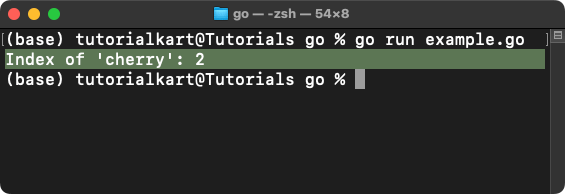
3. Find Index of First Element Matching a Condition
This example demonstrates how to find the index of the first element that matches a custom condition:
</>
Copy
package main
import "fmt"
// Function to find the index of the first element greater than a threshold
func findIndexCondition(slice []int, condition func(int) bool) int {
for i, v := range slice {
if condition(v) {
return i
}
}
return -1
}
func main() {
// Declare and initialize a slice of integers
numbers := []int{5, 10, 15, 20, 25}
// Find the index of the first number greater than 18
index := findIndexCondition(numbers, func(n int) bool {
return n > 18
})
// Print the result
fmt.Println("Index of first element > 18:", index)
}
Explanation
- Define Function: The
findIndexCondition
function takes a slice and a custom condition function as input. - Check Condition: The condition function determines whether each element satisfies the condition.
- Return Index: The function returns the index of the first element that satisfies the condition or
-1
if no such element is found. - Call Function: The slice
numbers
and a condition function checking for values greater than18
are passed to the function, and the index is printed.
Output
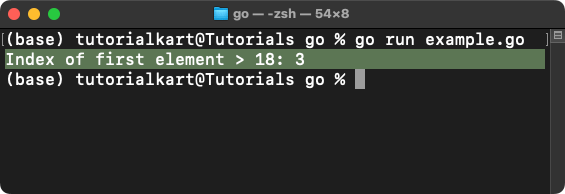
Points to Remember
- Custom Functions: Create custom functions to find indexes based on specific conditions.
- Linear Search: Finding an index involves a linear search through the slice, which has a time complexity of
O(n)
. - Handle Not Found: Return a sentinel value like
-1
if the target element is not found in the slice.