Go Slice – Get First Element
In Go, slices are dynamic collections that allow you to access elements by their index. To get the first element of a slice, you simply use the index 0
. However, you must ensure that the slice is not empty to avoid runtime errors.
In this tutorial, we will explore how to get the first element of a slice in Go with practical examples and detailed explanations.
Steps to Get the First Element of a Slice
- Check If Slice Is Empty: Ensure the slice has at least one element to avoid runtime errors.
- Access the First Element: Use the index
0
to retrieve the first element of the slice. - Handle Edge Cases: Return an error or a default value if the slice is empty.
Examples of Getting the First Element of a Slice
1 Get the First Element of a Slice of Integers
This example demonstrates how to get the first element of a slice of integers:
</>
Copy
package main
import "fmt"
// Function to get the first element of a slice
func getFirst(slice []int) (int, error) {
if len(slice) == 0 {
return 0, fmt.Errorf("slice is empty")
}
return slice[0], nil
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Get the first element
firstElement, err := getFirst(numbers)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the first element
fmt.Println("First Element:", firstElement)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns an error if true.
- Get First Element: The value at index
0
is retrieved and returned as the first element. - Print Result: The first element is printed in the main function.
Output
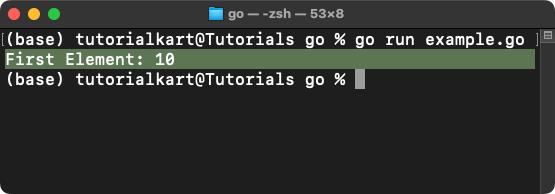
2 Get the First Element of a Slice of Strings
This example demonstrates how to get the first element of a slice of strings:
</>
Copy
package main
import "fmt"
// Function to get the first element of a slice
func getFirst(slice []string) (string, error) {
if len(slice) == 0 {
return "", fmt.Errorf("slice is empty")
}
return slice[0], nil
}
func main() {
// Declare and initialize a slice
words := []string{"apple", "banana", "cherry", "date"}
// Get the first element
firstElement, err := getFirst(words)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the first element
fmt.Println("First Element:", firstElement)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns an error if true.
- Get First Element: The value at index
0
is retrieved and returned as the first element. - Print Result: The first element is printed in the main function.
Output
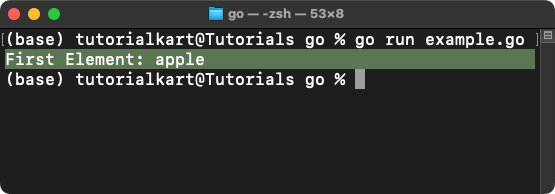
Points to Remember
- Bounds Checking: Always check if the slice is empty before accessing elements to prevent runtime errors.
- Generic Logic: The same logic can be applied to slices of any type, such as integers, strings, or custom structs.
- Default Values: When the slice is empty, return a default value (e.g.,
0
for integers or an empty string for strings) along with an error.