Go Slice – Get First N Elements
In Go, slices are dynamic collections that allow elements to be accessed using slicing operations. To get the first N
elements of a slice, you can use the slicing syntax slice[:N]
. However, you need to ensure that the slice has at least N
elements to avoid runtime errors.
In this tutorial, we will explore how to retrieve the first N
elements of a slice with practical examples and detailed explanations.
Steps to Get the First N Elements of a Slice
- Validate the Slice Length: Ensure the slice has at least
N
elements before slicing. - Use Slicing: Use the syntax
slice[:N]
to retrieve the firstN
elements. - Handle Edge Cases: If
N
is greater than the length of the slice, return the entire slice.
Examples of Getting the First N Elements of a Slice
1. Get the First N Elements from a Slice of Integers
This example demonstrates how to get the first N
elements from a slice of integers:
</>
Copy
package main
import "fmt"
// Function to get the first N elements of a slice
func getFirstN(slice []int, n int) []int {
if n > len(slice) {
return slice // Return the entire slice if n is greater than its length
}
return slice[:n]
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Get the first 3 elements
firstThree := getFirstN(numbers, 3)
// Print the result
fmt.Println("First 3 Elements:", firstThree)
}
Explanation
- Validate Input: The function checks if
n
is greater than the length of the slice and returns the entire slice if true. - Slicing: The slice is sliced using
slice[:n]
to retrieve the firstN
elements. - Print Result: The resulting slice is printed in the main function.
Output
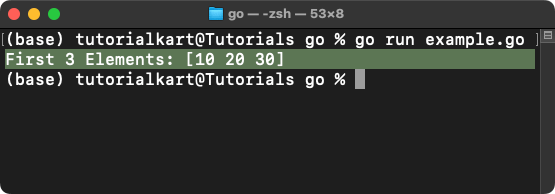
2. Get the First N Elements from a Slice of Strings
This example demonstrates how to get the first N
elements from a slice of strings:
</>
Copy
package main
import "fmt"
// Function to get the first N elements of a slice
func getFirstN(slice []string, n int) []string {
if n > len(slice) {
return slice // Return the entire slice if n is greater than its length
}
return slice[:n]
}
func main() {
// Declare and initialize a slice
words := []string{"apple", "banana", "cherry", "date", "elderberry"}
// Get the first 2 elements
firstTwo := getFirstN(words, 2)
// Print the result
fmt.Println("First 2 Elements:", firstTwo)
}
Explanation
- Validate Input: The function checks if
n
is greater than the length of the slice and returns the entire slice if true. - Slicing: The slice is sliced using
slice[:n]
to retrieve the firstN
elements. - Print Result: The resulting slice is printed in the main function.
Output
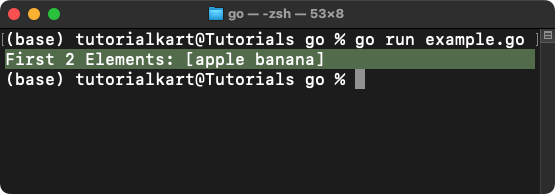
Points to Remember
- Slicing Syntax: Use
slice[:N]
to get the firstN
elements of a slice. - Bounds Handling: Ensure that
N
is not greater than the slice’s length, or handle cases where it is by returning the entire slice. - Generic Usage: The same logic can be applied to slices of any type, such as integers, strings, or custom structs.