Go Slice – Get Last Element
In Go, slices are dynamic collections that allow you to access elements by their index. To get the last element of a slice, you use the index len(slice) - 1
. However, you must ensure that the slice is not empty to avoid runtime errors.
In this tutorial, we will explore how to get the last element of a slice in Go with practical examples and detailed explanations.
Steps to Get the Last Element of a Slice
- Check If Slice Is Empty: Ensure the slice has at least one element to avoid runtime errors.
- Access the Last Element: Use the index
len(slice) - 1
to retrieve the last element of the slice. - Handle Edge Cases: Return an error or a default value if the slice is empty.
Examples of Getting the Last Element of a Slice
1 Get the Last Element of a Slice of Integers
This example demonstrates how to get the last element of a slice of integers:
</>
Copy
package main
import "fmt"
// Function to get the last element of a slice
func getLast(slice []int) (int, error) {
if len(slice) == 0 {
return 0, fmt.Errorf("slice is empty")
}
return slice[len(slice)-1], nil
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Get the last element
lastElement, err := getLast(numbers)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the last element
fmt.Println("Last Element:", lastElement)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns an error if true.
- Get Last Element: The value at index
len(slice) - 1
is retrieved and returned as the last element. - Print Result: The last element is printed in the main function.
Output
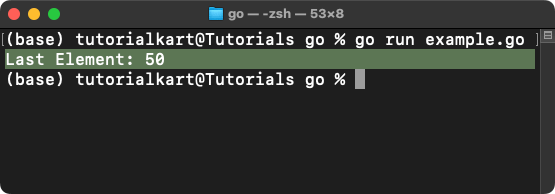
2 Get the Last Element of a Slice of Strings
This example demonstrates how to get the last element of a slice of strings:
</>
Copy
package main
import "fmt"
// Function to get the last element of a slice
func getLast(slice []string) (string, error) {
if len(slice) == 0 {
return "", fmt.Errorf("slice is empty")
}
return slice[len(slice)-1], nil
}
func main() {
// Declare and initialize a slice
words := []string{"apple", "banana", "cherry", "date"}
// Get the last element
lastElement, err := getLast(words)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the last element
fmt.Println("Last Element:", lastElement)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns an error if true.
- Get Last Element: The value at index
len(slice) - 1
is retrieved and returned as the last element. - Print Result: The last element is printed in the main function.
Output
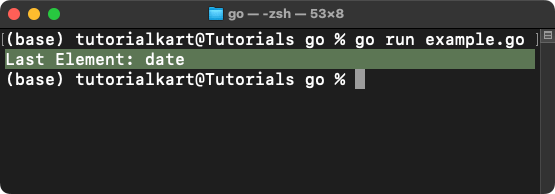
Points to Remember
- Bounds Checking: Always check if the slice is empty before accessing elements to prevent runtime errors.
- Generic Logic: The same logic can be applied to slices of any type, such as integers, strings, or custom structs.
- Default Values: When the slice is empty, return a default value (e.g.,
0
for integers or an empty string for strings) along with an error.