Go Slice – Insert Element
In Go, slices are dynamic and allow elements to be added or removed. However, inserting an element at a specific position in a slice requires manually creating a new slice to accommodate the new element. Go does not have a built-in function for slice insertion, but you can achieve this functionality with custom logic.
In this tutorial, we will explore how to insert an element into a slice at a specific index with practical examples and detailed explanations.
Steps to Insert an Element in a Slice
- Validate the Index: Ensure the insertion index is within the valid range of the slice.
- Split the Slice: Use slicing to split the original slice into two parts: before and after the insertion point.
- Append the Element: Insert the new element by combining the two parts with the new element in between.
Examples of Slice Element Insertion
1 Insert an Element at a Specific Index
This example demonstrates how to insert an element at a specific index in a slice:
</>
Copy
package main
import "fmt"
// Function to insert an element at a specific index in a slice
func insertElement(slice []int, index int, value int) []int {
if index < 0 || index > len(slice) {
fmt.Println("Invalid index")
return slice
}
// Insert the element
return append(slice[:index], append([]int{value}, slice[index:]...)...)
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Insert 25 at index 2
updatedSlice := insertElement(numbers, 2, 25)
// Print the updated slice
fmt.Println("Updated Slice:", updatedSlice)
}
Explanation
- Define Function: The
insertElement
function checks if the index is valid and uses slicing to insert the new element at the specified position. - Split Slice: The slice is split into two parts using
slice[:index]
andslice[index:]
. - Insert Element: The new element is inserted by appending it to the first part and then appending the second part.
- Print Result: The updated slice is printed in the main function.
Output
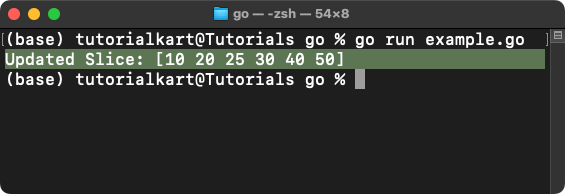
2 Insert an Element at the Beginning of a Slice
This example demonstrates how to insert an element at the beginning of a slice:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Insert 5 at the beginning
updatedSlice := append([]int{5}, numbers...)
// Print the updated slice
fmt.Println("Updated Slice:", updatedSlice)
}
Explanation
- Insert at Beginning: A new slice with the element
5
is created and concatenated with the original slice usingappend
. - Updated Slice: The resulting slice includes
5
as the first element, followed by the original elements.
Output
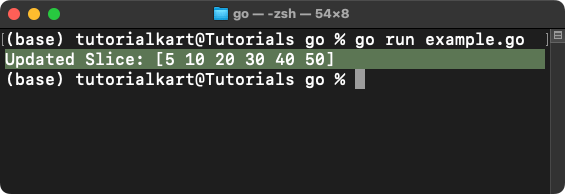
3 Insert an Element at the End of a Slice
This example demonstrates how to insert an element at the end of a slice:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Insert 60 at the end
updatedSlice := append(numbers, 60)
// Print the updated slice
fmt.Println("Updated Slice:", updatedSlice)
}
Explanation
- Insert at End: The element
60
is appended to the original slice usingappend
. - Updated Slice: The resulting slice includes
60
as the last element.
Output
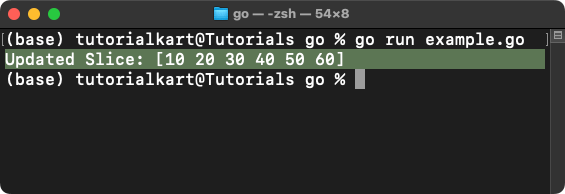
Points to Remember
- Valid Index: Ensure the index is within the range
[0, len(slice)]
to avoid errors. - Dynamic Slices: Use
append
for efficient slice operations. - Memory Allocation: Inserting elements may involve reallocating memory if the capacity of the slice is exceeded.