invalid argument: index must not be negative with Go Slices
In Go, attempting to access a slice with a negative index results in a compile-time error: invalid argument: index must not be negative
. This occurs because Go does not support negative indexing for slices, unlike some other programming languages.
In this tutorial, we will explore the cause of this error, demonstrate scenarios where it might occur, and provide strategies to avoid and handle it effectively.
What Causes the Error?
The error invalid argument: index must not be negative
is raised when:
- You attempt to access a slice element using a negative index (e.g.,
slice[-1]
). - You use a negative index when creating a subslice (e.g.,
slice[-1:len(slice)]
).
Unlike languages like Python that support negative indices to access elements from the end of a list, Go only allows zero-based positive indexing.
Examples of the Error
1. Accessing a Slice with a Negative Index
This example demonstrates how using a negative index to access a slice causes a compile-time error:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Attempt to access a negative index
fmt.Println(numbers[-1]) // This will cause a compile-time error
}
Error Output
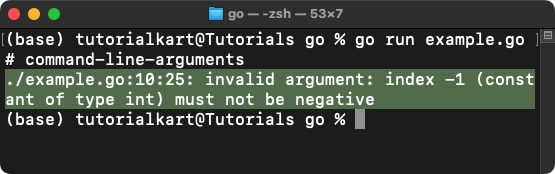
2. Creating a Subslice with a Negative Index
This example demonstrates how using a negative index in a subslice operation causes a compile-time error:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Attempt to create a subslice with a negative index
fmt.Println(numbers[-1:2]) // This will cause a compile-time error
}
Error Output
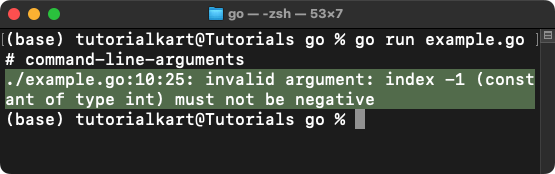
How to Prevent the Error
- Validate Indices: Always ensure indices are non-negative and within the slice’s valid range.
- Calculate Valid Indices: Use
len(slice)
to calculate indices dynamically if needed. - Simulate Negative Indices: If you need behavior similar to negative indexing, calculate the corresponding positive index manually (e.g.,
len(slice) - 1
for the last element).
Example of Simulating Negative Indexing
This example demonstrates how to simulate negative indexing in Go:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Simulate negative index to get the last element
negativeIndex := -1
if negativeIndex < 0 {
index := len(numbers) + negativeIndex
if index >= 0 && index < len(numbers) {
fmt.Println("Element at negative index:", numbers[index])
} else {
fmt.Println("Index out of range for negative index:", negativeIndex)
}
}
}
Output
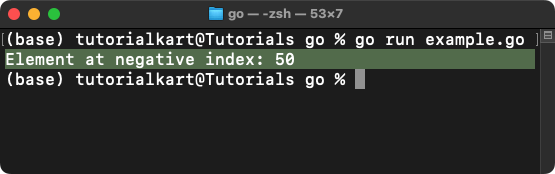
Points to Remember
- Go Does Not Support Negative Indices: Unlike Python, Go requires all slice indices to be non-negative.
- Simulate Negative Indexing: You can manually calculate indices using
len(slice)
for operations similar to negative indexing. - Always Validate Indices: Ensure indices are within the valid range of
0
tolen(slice)-1
before accessing slice elements.