Go – Iterate over Slice using Range
In Go, the range
keyword is used to iterate over slices, allowing you to access both the index and value of each element. This provides a concise and readable way to traverse and process slices.
In this tutorial, we will explore how to use range
with slices in Go, demonstrate practical examples, and provide detailed explanations.
Key Features of range
with Slices
- Index and Value: The
range
loop provides both the index and value of each element in the slice. - Index Only: You can ignore the value by using the blank identifier (
_
). - Value Only: You can ignore the index and retrieve only the value.
Examples of Using range
with Slices
1 Access Both Index and Value
This example demonstrates how to access both the index and value of each element in a slice:
</>
Copy
package main
import "fmt"
func main() {
// Define a slice
numbers := []int{10, 20, 30, 40, 50}
// Use range to iterate over the slice
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
}
Explanation
- Define Slice: A slice
numbers
is initialized with integers{10, 20, 30, 40, 50}
. - Iterate with Range: The
range
keyword is used to iterate over the slice, returning both the index and value of each element. - Print Results: The index and value are printed in each iteration using
fmt.Printf
.
Output
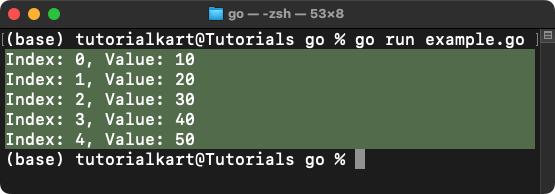
2 Access Only the Value
This example demonstrates how to access only the values in a slice:
</>
Copy
package main
import "fmt"
func main() {
// Define a slice
numbers := []int{5, 10, 15, 20, 25}
// Use range to iterate and access only the value
for _, value := range numbers {
fmt.Printf("Value: %d\n", value)
}
}
Explanation
- Define Slice: A slice
numbers
is initialized with integers{5, 10, 15, 20, 25}
. - Ignore Index: The blank identifier (
_
) is used to ignore the index returned byrange
. - Access Value: The
value
variable holds the current element in each iteration. - Print Results: The value is printed in each iteration using
fmt.Printf
.
Output
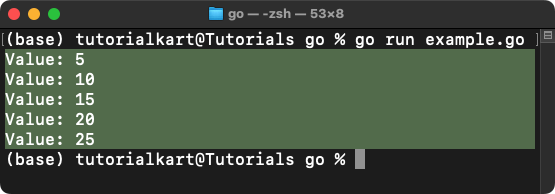
3 Access Only the Index
This example demonstrates how to access only the indices in a slice:
</>
Copy
package main
import "fmt"
func main() {
// Define a slice
numbers := []int{2, 4, 6, 8, 10}
// Use range to iterate and access only the index
for index := range numbers {
fmt.Printf("Index: %d\n", index)
}
}
Explanation
- Define Slice: A slice
numbers
is initialized with integers{2, 4, 6, 8, 10}
. - Ignore Value: The value returned by
range
is ignored by omitting it in the loop declaration. - Access Index: The
index
variable holds the current index in each iteration. - Print Results: The index is printed in each iteration using
fmt.Printf
.
Output
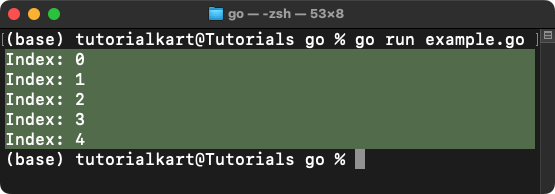
Points to Remember
- Index and Value: The
range
loop provides both the index and value for each element in a slice. - Blank Identifier: Use the blank identifier (
_
) to ignore either the index or value. - Immutable Values: Modifying the value variable in the loop does not change the slice. To update the slice, use the index directly.
- Flexible Syntax:
range
is versatile and works seamlessly with slices of any type.