Go Slice – Join Elements
In Go, joining elements of a slice into a single string is commonly done for slices of strings. This operation is often used when preparing data for display, logging, or file storage. The strings.Join
function in the strings
package is the most efficient way to join elements of a string slice.
In this tutorial, we will explore how to join slices in Go using the strings.Join
function and other approaches, with practical examples and explanations.
Syntax of strings.Join
The syntax for joining elements of a slice using strings.Join
is:
result := strings.Join(slice, separator)
Here:
slice
: The slice of strings to join.separator
: The string to insert between each element of the slice.result
: The resulting string after joining the elements.
Examples of Joining Slices
1 Join a Slice of Strings with a Space
This example demonstrates how to join elements of a slice into a single string separated by a space:
package main
import (
"fmt"
"strings"
)
func main() {
// Declare and initialize a slice of strings
words := []string{"Go", "is", "awesome"}
// Join the elements with a space
result := strings.Join(words, " ")
// Print the resulting string
fmt.Println("Joined String:", result)
}
Explanation
- Declare Slice: The slice
words
is initialized with values{"Go", "is", "awesome"}
. - Join Elements: The
strings.Join
function concatenates the elements with a space (" "
) as the separator. - Print Result: The resulting string
"Go is awesome"
is printed usingfmt.Println
.
Output
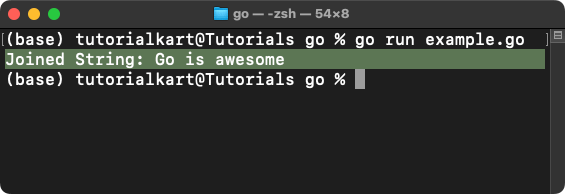
2 Join a Slice of Strings with a Comma
This example demonstrates how to join elements of a slice with a comma:
package main
import (
"fmt"
"strings"
)
func main() {
// Declare and initialize a slice of strings
fruits := []string{"apple", "banana", "cherry"}
// Join the elements with a comma
result := strings.Join(fruits, ", ")
// Print the resulting string
fmt.Println("Joined String:", result)
}
Explanation
- Declare Slice: The slice
fruits
is initialized with values{"apple", "banana", "cherry"}
. - Join Elements: The
strings.Join
function concatenates the elements with a comma and space (", "
) as the separator. - Print Result: The resulting string
"apple, banana, cherry"
is printed usingfmt.Println
.
Output
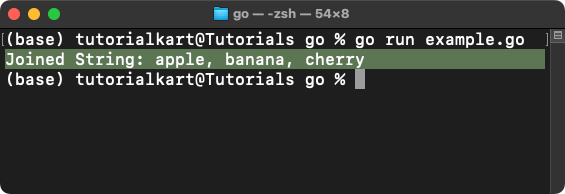
3 Custom Join Without strings.Join
This example demonstrates how to manually join elements of a slice without using strings.Join
:
package main
import "fmt"
func main() {
// Declare and initialize a slice of strings
words := []string{"Hello", "World", "Go"}
// Manually join elements with a dash
result := ""
for i, word := range words {
result += word
if i < len(words)-1 {
result += "-"
}
}
// Print the resulting string
fmt.Println("Joined String:", result)
}
Explanation
- Initialize Result: An empty string
result
is initialized to store the joined string. - Iterate Through Slice: A
for
loop iterates through the slice, adding each word to the result string. - Add Separator: A dash (
"-"
) is added after each word except the last one. - Print Result: The resulting string
"Hello-World-Go"
is printed usingfmt.Println
.
Output
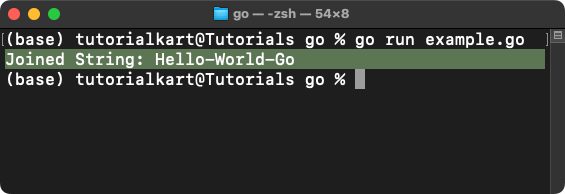
Points to Remember
- Efficient Joining: Use
strings.Join
for efficient joining of string slices. - Separator Choice: Choose an appropriate separator based on the context (e.g., space, comma, or custom characters).
- Custom Joining: For more control, manually iterate through the slice and construct the result string.