Go Slice Merge
In Go, merging slices refers to combining multiple slices into a single slice. This operation is commonly performed using the built-in append()
function. Merging slices is efficient and straightforward, allowing you to work with dynamic collections of data.
In this tutorial, we will explore how to merge slices in Go with practical examples and detailed explanations.
Steps to Merge Slices
- Create the Slices: Define the slices you want to merge.
- Use
append()
: Use theappend()
function to combine the slices into a single slice. - Handle Empty Slices: Ensure that merging works even if one or more slices are empty.
Examples of Merging Slices
1. Merge Two Slices of Integers
This example demonstrates how to merge two slices of integers:
</>
Copy
package main
import "fmt"
func main() {
// Define two slices
slice1 := []int{10, 20, 30}
slice2 := []int{40, 50, 60}
// Merge the slices
merged := append(slice1, slice2...)
// Print the result
fmt.Println("Slice1:", slice1)
fmt.Println("Slice2:", slice2)
fmt.Println("Merged Slice:", merged)
}
Explanation
- Create Slices: Two slices,
slice1
andslice2
, are defined with integer elements. - Merge Slices: The
append()
function is used to mergeslice2
intoslice1
. The...
operator unpacks the elements ofslice2
. - Print Results: The original slices and the merged slice are printed to verify the operation.
Output
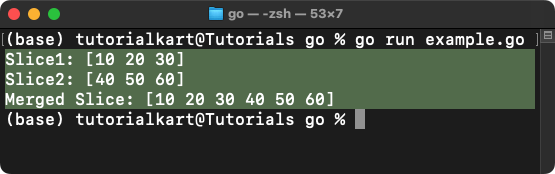
2. Merge Multiple Slices of Strings
This example demonstrates how to merge multiple slices of strings:
</>
Copy
package main
import "fmt"
func main() {
// Define multiple slices
slice1 := []string{"apple", "banana"}
slice2 := []string{"cherry", "date"}
slice3 := []string{"elderberry", "fig"}
// Merge the slices
merged := append(slice1, append(slice2, slice3...)...)
// Print the result
fmt.Println("Slice1:", slice1)
fmt.Println("Slice2:", slice2)
fmt.Println("Slice3:", slice3)
fmt.Println("Merged Slice:", merged)
}
Explanation
- Create Slices: Three slices,
slice1
,slice2
, andslice3
, are defined with string elements. - Merge Slices: The
append()
function is used twice: first to mergeslice2
andslice3
, and then to merge the result withslice1
. - Print Results: The original slices and the merged slice are printed to verify the operation.
Output
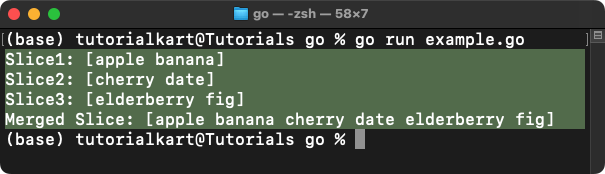
Points to Remember
- Efficient Merging: Use the
append()
function with the...
operator to merge slices efficiently. - Handle Empty Slices: Merging an empty slice has no effect and does not raise an error.
- Data Integrity: The original slices remain unchanged during the merging process.
- Flexible Operation: You can merge slices of any type, such as integers, strings, or structs, using the same technique.