Go Slice – Negative Index
In many programming languages like Python, negative indexing allows you to access elements of a collection from the end, where -1
represents the last element, -2
represents the second last, and so on. However, Go does not natively support negative indexing for slices. Attempting to use a negative index directly will result in a compilation error.
In this tutorial, we will discuss how to simulate negative indexing in Go by using variables and calculations to access elements from the end of a slice.
Why Go Does Not Support Negative Indexing
Go enforces simplicity and safety by strictly checking index bounds for slices. Negative indexing, while convenient, could introduce complexity and ambiguous behavior in Go’s design principles. Instead, Go encourages explicit handling of indices to ensure clarity and prevent errors.
Simulating Negative Indexing in Go
- Calculate Index: Use a variable to represent the negative index, and calculate the corresponding positive index using
len(slice) + negativeIndex
. - Access Element: Use the calculated positive index to access elements from the end of the slice.
Examples of Simulating Negative Indexing
1. Access Elements Using a Negative Index Variable
This example demonstrates how to use a negative index variable to access elements from the end of a slice:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Use a negative index variable
negativeIndex := -1
last := numbers[len(numbers)+negativeIndex]
// Use another negative index variable
negativeIndex = -2
secondLast := numbers[len(numbers)+negativeIndex]
// Print the results
fmt.Println("Last Element:", last)
fmt.Println("Second Last Element:", secondLast)
}
Explanation
- Negative Index Variable: The variable
negativeIndex
is assigned-1
to access the last element and-2
to access the second last element. - Calculate Positive Index: The positive index is calculated using
len(numbers) + negativeIndex
. - Access Elements: The elements at the calculated indices are retrieved from the slice.
- Print Results: The retrieved elements are printed using
fmt.Println
.
Output
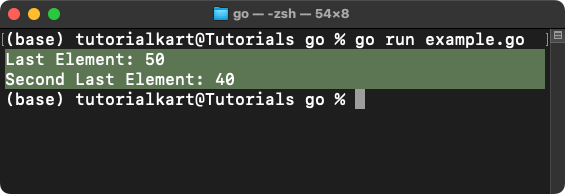
2. Simulating Negative Indexing with a Function
This example demonstrates how to use a custom function to simulate negative indexing:
package main
import "fmt"
// Function to simulate negative indexing
func getElement(slice []int, negativeIndex int) (int, error) {
if negativeIndex >= 0 || -negativeIndex > len(slice) {
return 0, fmt.Errorf("invalid negative index")
}
return slice[len(slice)+negativeIndex], nil
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Get the last element using negative index
last, _ := getElement(numbers, -1)
// Get the second last element using negative index
secondLast, _ := getElement(numbers, -2)
// Print the results
fmt.Println("Last Element:", last)
fmt.Println("Second Last Element:", secondLast)
}
Explanation
- Define Function: The
getElement
function calculates the positive index equivalent of a negative index usinglen(slice) + negativeIndex
. - Validate Index: The function checks if the negative index is valid.
- Access Element: The element is accessed using the calculated index and returned.
- Call Function: The
getElement
function is called with-1
and-2
to retrieve the last and second last elements, respectively.
Output
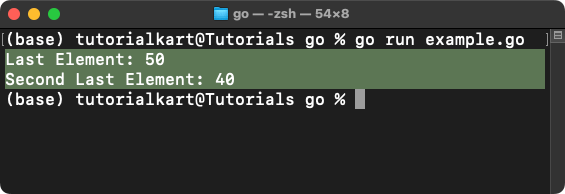
Points to Remember
- No Built-In Support: Go does not support negative indexing for slices, unlike some other languages.
- Use Variables: Use a variable to represent negative indices and calculate positive indices dynamically.
- Custom Functions: Create utility functions to simulate negative indexing for cleaner and reusable code.
- Bounds Checking: Always validate indices to avoid runtime errors.