panic: runtime error: index out of range with Go Slices
In Go, slices provide dynamic access to a collection of elements, but working with slices outside their valid bounds can result in the runtime error: panic: runtime error: index out of range
. This error occurs when you try to access or manipulate an index that is invalid for the given slice.
In this tutorial, we will explore what causes this error, provide examples of common scenarios, and discuss how to prevent and handle this runtime error effectively.
What Causes Index Out of Range Error?
The index out of range
error occurs when you try to access an index that is greater than or equal to the length of the slice.
Examples of Index Out of Range Error
1. Accessing an Invalid Index
This example demonstrates how accessing an index outside the valid range of a slice results in an error:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Attempt to access an invalid index
fmt.Println(numbers[3]) // This will cause a panic
}
Output
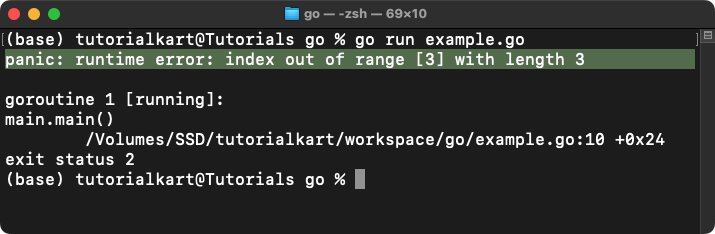
How to Prevent Index Out of Range Error
- Check Slice Length: Always use
len(slice)
to validate indices before accessing or slicing. - Use Conditional Statements: Ensure that indices are within the valid range of
0
tolen(slice)-1
. - Handle Edge Cases: Consider scenarios where the slice might be empty or smaller than expected.
Example of Preventing Index Out of Range Error
Safe Access with Index Validation
This example demonstrates how to safely access a slice element using index validation:
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Safely access an index
index := 3
if index >= 0 && index < len(numbers) {
fmt.Println("Element at index", index, ":", numbers[index])
} else {
fmt.Println("Index out of range:", index)
}
}
Output
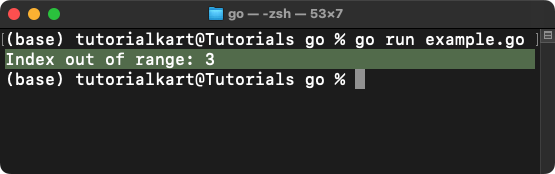
Points to Remember
- Validate Indices: Always ensure that indices are within the valid range of the slice before accessing elements or creating subslices.
- Check Slice Length: Use
len(slice)
to determine the valid range of indices. - Handle Edge Cases: Ensure your code gracefully handles empty or unexpectedly short slices.
- Defensive Programming: Implement checks to prevent runtime errors and ensure robust code.