Go Slice Pointer
In Go, slices are built on top of arrays and consist of three components: a pointer to the underlying array, the slice’s length, and its capacity. The pointer allows slices to reference and share the same underlying data without copying it.
In this tutorial, we will explore the concept of slice pointers, demonstrate practical examples, and explain how slices share and manipulate underlying data.
Key Concepts of Slice Pointers
- Pointer to Array: A slice points to the memory location of the first element in its underlying array.
- Shared Data: Multiple slices can reference the same array, allowing changes in one slice to reflect in others.
- Independent Metadata: Each slice maintains its own length and capacity, even if they share the same array.
Examples of Slice Pointers
1. Single Slice Sharing Array
This example demonstrates how a slice references the underlying array:
</>
Copy
package main
import "fmt"
func main() {
// Declare an array
arr := [5]int{10, 20, 30, 40, 50}
// Create a slice from the array
slice := arr[1:4]
// Modify the slice
slice[0] = 99
// Print the array and slice
fmt.Println("Array after modification:", arr)
fmt.Println("Slice after modification:", slice)
}
Explanation
- Array Declaration: An array
arr
is initialized with five integers. - Slice Creation: A slice
slice
is created from indices1
to4
, pointing to the corresponding segment of the array. - Modify Slice: Modifying the first element of
slice
(index1
ofarr
) updates the underlying array. - Shared Data: Since the slice shares its underlying data with the array, changes in the slice reflect in the array.
Output
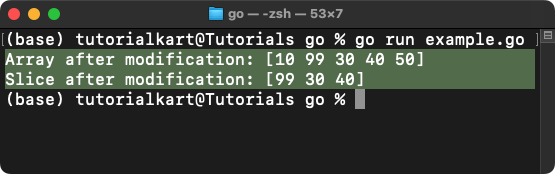
2. Multiple Slices Sharing an Array
This example demonstrates how multiple slices can reference the same underlying array:
</>
Copy
package main
import "fmt"
func main() {
// Declare an array
arr := [5]int{10, 20, 30, 40, 50}
// Create two slices referencing the same array
slice1 := arr[1:4]
slice2 := arr[2:5]
// Modify the first slice
slice1[1] = 99
// Print the array and both slices
fmt.Println("Array after modification:", arr)
fmt.Println("Slice1 after modification:", slice1)
fmt.Println("Slice2 after modification:", slice2)
}
Explanation
- Array Declaration: An array
arr
is initialized with five integers. - Create Two Slices: Two slices
slice1
andslice2
are created, referencing overlapping parts ofarr
. - Modify Slice1: Modifying
slice1[1]
updates the underlying array, which affectsslice2
as well. - Shared Data: Both slices share the same array, so changes in one slice are visible in the other.
Output
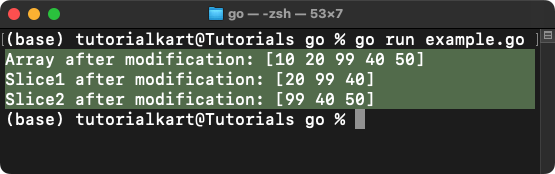
Points to Remember
- Shared Data: Slices share the same underlying array, allowing efficient data manipulation but introducing interdependence.
- Independent Metadata: Each slice maintains its own length and capacity, even if they share the same array.
- Pointer Behavior: Modifications in a slice reflect in all other slices referencing the same array.
- Safe Manipulation: Use
copy()
to create independent slices if you need to avoid unintended side effects.