Go Slice Pop
In Go, slices are dynamic data structures that allow elements to be added or removed. While Go does not have built-in functions for operations like “pop” (removing the last element), you can implement this functionality by manipulating the slice using slicing operations.
In this tutorial, we will explore how to implement the “pop” operation to remove the last element or the first element from a slice, with practical examples and detailed explanations.
What is a Pop Operation?
The “pop” operation refers to removing an element from a collection, typically from the end (last element) or the beginning (first element). After removing the element, the remaining elements are preserved, and the size of the collection is reduced.
Implementing Slice Pop in Go
- Remove Last Element: Use slicing to exclude the last element from the slice, i.e.,
slice[:len(slice)-1]
. - Remove First Element: Use slicing to exclude the first element from the slice, i.e.,
slice[1:]
. - Handle Empty Slices: Check if the slice is empty to avoid runtime errors.
Examples of Slice Pop
1 Pop the Last Element
This example demonstrates how to remove the last element from a slice:
package main
import "fmt"
// Function to pop the last element from a slice
func popLast(slice []int) ([]int, int, error) {
if len(slice) == 0 {
return nil, 0, fmt.Errorf("slice is empty")
}
// Get the last element
lastElement := slice[len(slice)-1]
// Return the new slice without the last element and the popped element
return slice[:len(slice)-1], lastElement, nil
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Pop the last element
updatedSlice, poppedElement, err := popLast(numbers)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the results
fmt.Println("Updated Slice:", updatedSlice)
fmt.Println("Popped Element:", poppedElement)
}
Explanation
- Define Function: The
popLast
function checks if the slice is empty and retrieves the last element. - Remove Last Element: The slice is sliced up to
len(slice)-1
to exclude the last element. - Return Results: The updated slice and the removed element are returned.
- Print Results: The updated slice and popped element are printed in the main function.
Output
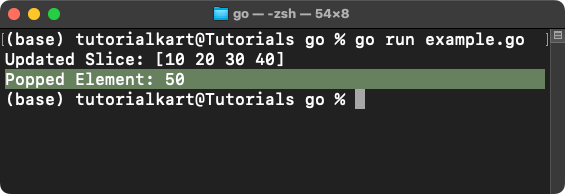
2 Pop the First Element
This example demonstrates how to remove the first element from a slice:
package main
import "fmt"
// Function to pop the first element from a slice
func popFirst(slice []int) ([]int, int, error) {
if len(slice) == 0 {
return nil, 0, fmt.Errorf("slice is empty")
}
// Get the first element
firstElement := slice[0]
// Return the new slice without the first element and the popped element
return slice[1:], firstElement, nil
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Pop the first element
updatedSlice, poppedElement, err := popFirst(numbers)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the results
fmt.Println("Updated Slice:", updatedSlice)
fmt.Println("Popped Element:", poppedElement)
}
Explanation
- Define Function: The
popFirst
function checks if the slice is empty and retrieves the first element. - Remove First Element: The slice is sliced from index
1
to exclude the first element. - Return Results: The updated slice and the removed element are returned.
- Print Results: The updated slice and popped element are printed in the main function.
Output
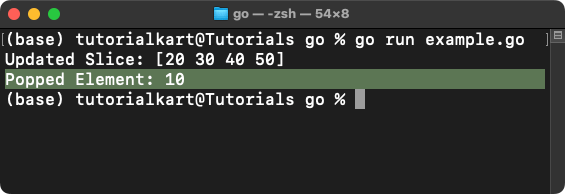
Points to Remember
- No Built-In Functionality: Go does not provide built-in pop operations for slices.
- Use Slicing: Use slicing operations to remove elements from the beginning or end of a slice.
- Check for Empty Slices: Always validate that the slice is not empty to avoid runtime errors.