Go Slice Prepend
In Go, prepending to a slice involves adding an element or elements to the beginning of the slice. While Go does not have a built-in method for prepending, you can achieve it by creating a new slice and using the append()
function.
In this tutorial, we will explore how to prepend elements to a slice in Go, demonstrate practical examples, and provide detailed explanations.
Steps to Prepend to a Slice
- Create a New Slice: Define a new slice that will hold the prepended elements.
- Use
append()
: Append the original slice to the new slice containing the prepended elements. - Reassign the Slice: Assign the result back to the original slice variable if needed.
Examples of Prepending to a Slice
1 Prepend a Single Element
This example demonstrates how to prepend a single element to a slice:
</>
Copy
package main
import "fmt"
func main() {
// Original slice
slice := []int{20, 30, 40}
// Prepend an element
slice = append([]int{10}, slice...)
// Print the result
fmt.Println("Prepended Slice:", slice)
}
Explanation
- Create Original Slice: The slice
slice
is initialized with integers{20, 30, 40}
. - Create New Slice: A new slice containing the element
10
is created inline using[]int{10}
. - Use
append()
: Theappend()
function combines the new slice with the original slice, prepending the element. - Reassign the Slice: The result is reassigned to
slice
.
Output
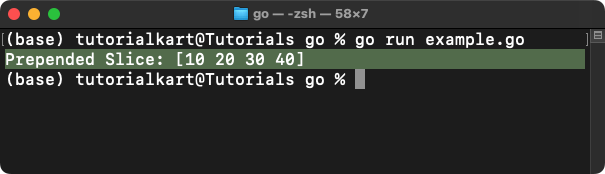
2 Prepend Multiple Elements
This example demonstrates how to prepend multiple elements to a slice:
</>
Copy
package main
import "fmt"
func main() {
// Original slice
slice := []int{30, 40, 50}
// Prepend multiple elements
slice = append([]int{10, 20}, slice...)
// Print the result
fmt.Println("Prepended Slice:", slice)
}
Explanation
- Create Original Slice: The slice
slice
is initialized with integers{30, 40, 50}
. - Create New Slice: A new slice containing the elements
{10, 20}
is created inline using[]int{10, 20}
. - Use
append()
: Theappend()
function combines the new slice with the original slice, prepending the elements. - Reassign the Slice: The result is reassigned to
slice
.
Output
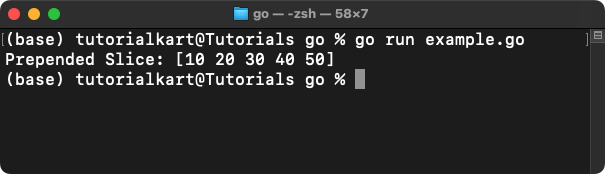
Points to Remember
- Efficient Prepending: Prepending in Go involves creating a new slice and combining it with the original slice.
- Use
append()
: Theappend()
function with the...
operator simplifies the prepending process. - Memory Considerations: Prepending creates a new slice, so memory allocation is involved.
- Flexible Operation: The same technique works for slices of any type, such as integers, strings, or structs.