Go – Slice Remove Duplicates
In Go, slices are dynamic collections that can contain duplicate elements. Removing duplicates involves creating a new slice that includes only unique elements from the original slice. Go does not provide a built-in function for this, so you need to implement custom logic using maps or loops.
In this tutorial, we will explore how to remove duplicate elements from a slice with practical examples and detailed explanations.
Steps to Remove Duplicates from a Slice
- Initialize a Map: Use a map to track elements that have already been added to the new slice.
- Iterate Through the Slice: Use a loop to check if an element exists in the map.
- Append Unique Elements: Add elements that are not in the map to the new slice and update the map.
Examples of Removing Duplicates from a Slice
1. Remove Duplicates from a Slice of Integers
This example demonstrates how to remove duplicates from a slice of integers using a map:
</>
Copy
package main
import "fmt"
// Function to remove duplicates from a slice of integers
func removeDuplicates(slice []int) []int {
uniqueMap := make(map[int]bool)
result := []int{}
for _, v := range slice {
if !uniqueMap[v] {
uniqueMap[v] = true
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize a slice with duplicates
numbers := []int{10, 20, 20, 30, 40, 40, 50}
// Remove duplicates
uniqueNumbers := removeDuplicates(numbers)
// Print the result
fmt.Println("Original Slice:", numbers)
fmt.Println("Slice without Duplicates:", uniqueNumbers)
}
Explanation
- Initialize Map: A map
uniqueMap
is used to track elements that have already been added to the result slice. - Iterate Through Slice: The loop checks if an element exists in the map. If it doesn’t, the element is added to both the map and the result slice.
- Return Result: The new slice containing only unique elements is returned.
- Print Results: The original and updated slices are printed in the main function.
Output
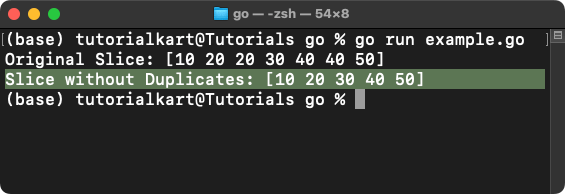
2. Remove Duplicates from a Slice of Strings
This example demonstrates how to remove duplicates from a slice of strings:
</>
Copy
package main
import "fmt"
// Function to remove duplicates from a slice of strings
func removeDuplicates(slice []string) []string {
uniqueMap := make(map[string]bool)
result := []string{}
for _, v := range slice {
if !uniqueMap[v] {
uniqueMap[v] = true
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize a slice with duplicates
words := []string{"apple", "banana", "apple", "cherry", "banana", "date"}
// Remove duplicates
uniqueWords := removeDuplicates(words)
// Print the result
fmt.Println("Original Slice:", words)
fmt.Println("Slice without Duplicates:", uniqueWords)
}
Explanation
- Initialize Map: A map
uniqueMap
is used to track strings that have already been added to the result slice. - Iterate Through Slice: The loop checks if a string exists in the map. If it doesn’t, the string is added to both the map and the result slice.
- Return Result: The new slice containing only unique strings is returned.
- Print Results: The original and updated slices are printed in the main function.
Output
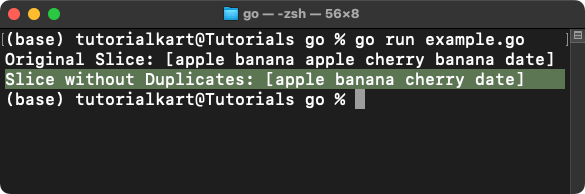
Points to Remember
- Use Maps: Maps are efficient for tracking unique elements in a slice, as they provide O(1) lookup time.
- Generic Logic: The same logic can be applied to slices of any type, such as integers, strings, or structs, by modifying the map key type.
- Preserve Order: The examples above preserve the original order of elements in the slice.
- Memory Usage: Removing duplicates requires additional memory for the map and the result slice.