Go Slice – Remove Element
In Go, slices are dynamic collections that allow elements to be added or removed. Removing an element from a slice requires creating a new slice that excludes the element you want to remove. Go does not provide a built-in function to remove an element, but you can achieve this using slicing operations.
In this tutorial, we will explore how to remove an element from a slice by index or by value, with practical examples and detailed explanations.
Steps to Remove an Element from a Slice
- Remove by Index: Use slicing to exclude the element at the specified index.
- Remove by Value: Iterate through the slice, adding elements to a new slice except for the one to remove.
- Handle Edge Cases: Ensure the index is valid or handle cases where the value is not found.
Examples of Removing an Element from a Slice
1 Remove an Element by Index
This example demonstrates how to remove an element from a slice by its index:
</>
Copy
package main
import "fmt"
// Function to remove an element by index
func removeByIndex(slice []int, index int) ([]int, error) {
if index < 0 || index >= len(slice) {
return nil, fmt.Errorf("index out of bounds")
}
return append(slice[:index], slice[index+1:]...), nil
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
fmt.Println("Original Slice:", numbers)
// Remove the element at index 2
updatedSlice, err := removeByIndex(numbers, 2)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the updated slice
fmt.Println("Updated Slice:", updatedSlice)
}
Explanation
- Validate Index: The function checks if the index is within bounds to avoid runtime errors.
- Remove Element: The element at the specified index is excluded by slicing the slice into two parts and appending them.
- Return Updated Slice: The modified slice is returned to the caller.
- Print Results: The original and updated slices are printed to show the result.
Output
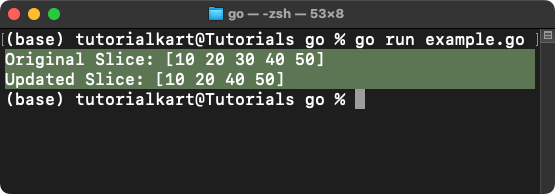
2 Remove an Element by Value
This example demonstrates how to remove an element from a slice by its value:
</>
Copy
package main
import "fmt"
// Function to remove an element by value
func removeByValue(slice []int, value int) []int {
result := []int{}
for _, v := range slice {
if v != value {
result = append(result, v)
}
}
return result
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Remove the value 30
updatedSlice := removeByValue(numbers, 30)
// Print the updated slice
fmt.Println("Original Slice:", numbers)
fmt.Println("Updated Slice:", updatedSlice)
}
Explanation
- Iterate Through Slice: The function iterates through the slice, checking each element against the value to remove.
- Append Unique Elements: Elements that do not match the value are appended to a new slice.
- Return Updated Slice: The new slice containing only the desired elements is returned.
- Print Results: The original and updated slices are printed to show the result.
Output
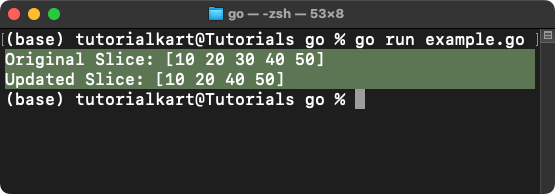
Points to Remember
- Index Validation: Always validate the index when removing an element by index to avoid runtime errors.
- Efficiency: Removing by index is more efficient than removing by value as it avoids iteration over the entire slice.
- Immutable Results: Removing by value creates a new slice, leaving the original slice unchanged.
- Use Cases: Choose the appropriate method (index or value) based on your application’s requirements.