Go Slice – Remove Last Element
In Go, slices are dynamic collections that allow elements to be added or removed. Removing the last element from a slice can be easily achieved using slicing operations. This operation creates a new slice that excludes the last element and references the remaining elements of the original slice.
In this tutorial, we will explore how to remove the last element from a slice with practical examples and detailed explanations.
Steps to Remove the Last Element from a Slice
- Validate the Slice: Ensure the slice is not empty before attempting to remove the last element.
- Use Slicing: Exclude the last element by slicing the original slice up to
len(slice) - 1
. - Handle Edge Cases: Return an empty slice if the original slice has only one element.
Examples of Removing the Last Element from a Slice
1. Remove the Last Element from a Slice of Integers
This example demonstrates how to remove the last element from a slice of integers:
</>
Copy
package main
import "fmt"
// Function to remove the last element from a slice
func removeLast(slice []int) []int {
if len(slice) == 0 {
return slice // Return the original slice if it's empty
}
return slice[:len(slice)-1]
}
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Remove the last element
updatedSlice := removeLast(numbers)
// Print the original and updated slices
fmt.Println("Original Slice:", numbers)
fmt.Println("Updated Slice:", updatedSlice)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns it unchanged if true.
- Use Slicing: The slice is sliced up to
len(slice)-1
to exclude the last element. - Return Updated Slice: The updated slice is returned, excluding the last element.
- Print Results: The original and updated slices are printed to demonstrate the result.
Output
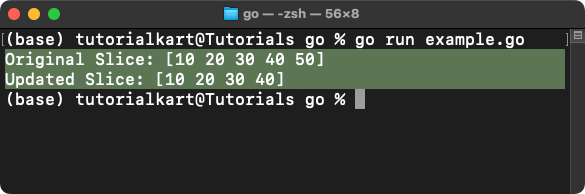
2. Remove the Last Element from a Slice of Strings
This example demonstrates how to remove the last element from a slice of strings:
</>
Copy
package main
import "fmt"
// Function to remove the last element from a slice
func removeLast(slice []string) []string {
if len(slice) == 0 {
return slice // Return the original slice if it's empty
}
return slice[:len(slice)-1]
}
func main() {
// Declare and initialize a slice
words := []string{"apple", "banana", "cherry", "date"}
// Remove the last element
updatedSlice := removeLast(words)
// Print the original and updated slices
fmt.Println("Original Slice:", words)
fmt.Println("Updated Slice:", updatedSlice)
}
Explanation
- Validate Slice: The function checks if the slice is empty and returns it unchanged if true.
- Use Slicing: The slice is sliced up to
len(slice)-1
to exclude the last element. - Return Updated Slice: The updated slice is returned, excluding the last element.
- Print Results: The original and updated slices are printed to demonstrate the result.
Output
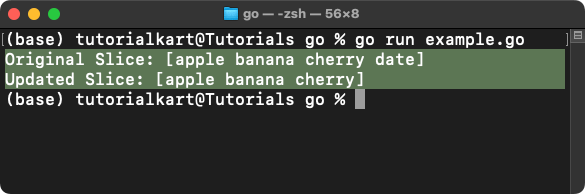
Points to Remember
- Empty Slice Handling: Always check if the slice is empty before attempting to remove elements.
- Memory Efficiency: The new slice references the same underlying array as the original slice, so changes to the new slice may affect the original.
- Edge Cases: If the slice has only one element, slicing will return an empty slice.
- Generic Logic: The same logic can be applied to slices of any type, such as integers, strings, or custom structs.