Go Slice – Resize
In Go, slices are dynamic collections that can grow or shrink by creating new slices based on the existing one. Resizing a slice involves either truncating it to reduce its length or expanding it to add new elements. Go provides simple slicing operations and functions like append
to resize slices.
In this tutorial, we will learn how to resize slices in Go with practical examples and detailed explanations.
Steps to Resize a Slice
- Truncate a Slice: Use slicing to reduce the length of a slice, e.g.,
slice[:newLength]
. - Expand a Slice: Use the
append
function to add new elements and increase the slice’s size. - Preserve Data: Ensure that resizing operations retain or discard elements as needed.
Examples of Resizing a Slice
1 Truncate a Slice
This example demonstrates how to truncate a slice by reducing its length:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Truncate the slice to length 3
truncatedSlice := numbers[:3]
// Print the original and truncated slices
fmt.Println("Original Slice:", numbers)
fmt.Println("Truncated Slice:", truncatedSlice)
}
Explanation
- Original Slice: The slice
numbers
is initialized with five elements. - Truncate Slice: The slice is resized to a length of 3 using
numbers[:3]
, which keeps only the first three elements. - Print Results: The original and truncated slices are printed to show the result.
Output
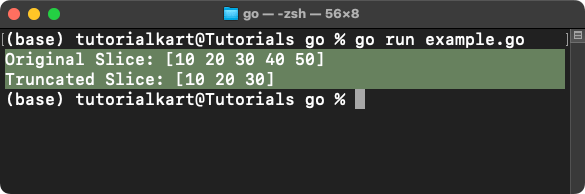
2 Expand a Slice
This example demonstrates how to expand a slice by adding new elements:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30}
// Expand the slice by adding elements
expandedSlice := append(numbers, 40, 50, 60)
// Print the original and expanded slices
fmt.Println("Original Slice:", numbers)
fmt.Println("Expanded Slice:", expandedSlice)
}
Explanation
- Original Slice: The slice
numbers
is initialized with three elements. - Expand Slice: The
append
function adds new elements40
,50
, and60
to the slice. - Print Results: The original and expanded slices are printed to show the result.
Output
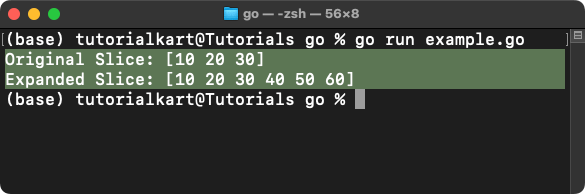
3 Resize a Slice Dynamically
This example demonstrates how to dynamically resize a slice using both truncation and expansion:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
numbers := []int{10, 20, 30, 40, 50}
// Truncate the slice to length 3
truncatedSlice := numbers[:3]
// Expand the slice by adding new elements
resizedSlice := append(truncatedSlice, 60, 70)
// Print the original, truncated, and resized slices
fmt.Println("Original Slice:", numbers)
fmt.Println("Truncated Slice:", truncatedSlice)
fmt.Println("Resized Slice:", resizedSlice)
}
Explanation
- Original Slice: The slice
numbers
is initialized with five elements. - Truncate Slice: The slice is resized to a length of 3 using
numbers[:3]
. - Expand Slice: The
append
function adds new elements60
and70
to the truncated slice. - Print Results: The original, truncated, and resized slices are printed to show the results.
Output
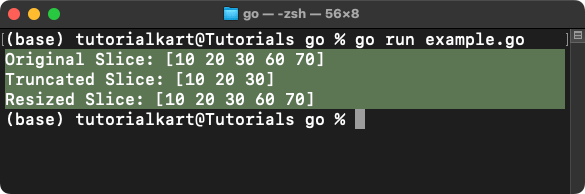
Points to Remember
- Memory Sharing: Truncated slices share memory with the original slice, so changes to the truncated slice may affect the original.
- Capacity Growth: When expanding a slice, if its capacity is exceeded, Go allocates a new underlying array to accommodate the additional elements.
- Edge Cases: Always check for empty slices to avoid runtime errors when truncating or expanding.
- Dynamic Resizing: Combining truncation and expansion allows flexible resizing of slices in Go.